exception handling
What is exception handling?
Exception handling is the process of responding to unwanted or unexpected events when a computer program runs. Exception handling deals with these events to avoid the program or system crashing, and without this process, exceptions would disrupt the normal operation of a program.
Exceptions occur for numerous reasons, including invalid user input, code errors, device failure, the loss of a network connection, insufficient memory to run an application, a memory conflict with another program, a program attempting to divide by zero or a user attempting to open files that are unavailable.
When an exception occurs, specialized programming language constructs, interrupt hardware mechanisms or operating system interprocess communication facilities handle the exception.
Exception handling differs from error handling in that the former involves conditions an application might catch versus serious problems an application might want to avoid. In contrast, error handling helps maintain the normal flow of software program execution.
How is exception handling used?
If a program has a lot of statements and an exception happens halfway through its execution, the statements after the exception do not execute, and the program crashes. Exception handling helps ensure this does not happen when an exception occurs.
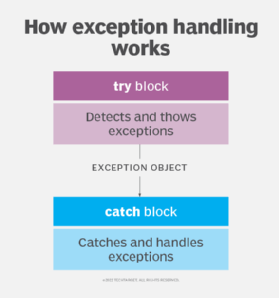
Exception handling can catch and throw exceptions. If a detecting function in a block of code cannot deal with an anomaly, the exception is thrown to a function that can handle the exception. A catch statement is a group of statements that handle the specific thrown exception. Catch parameters determine the specific type of exception that is thrown.
Exception handling is useful for dealing with exceptions that cannot be handled locally. Instead of showing an error status in the program, the exception handler transfers control to where the error can be handled. A function can throw exceptions or can choose to handle exceptions.
Error handling code can also be separated from normal code with the use of try blocks, which is code that is enclosed in curly braces or brackets that could cause an exception. Try blocks can help programmers to categorize exception objects.
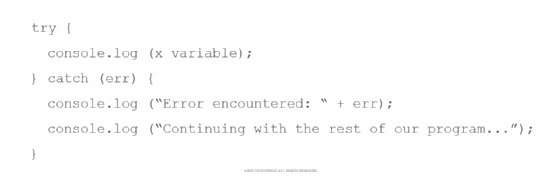
What are the types of exceptions?
Exceptions can come in the following two exception classes:
- Checked exceptions. Also called compile-time exceptions, the compiler checks these exceptions during the compilation process to confirm if the exception is being handled by the programmer. If not, then a compilation error displays on the system. Checked exceptions include SQLException and ClassNotFoundException.
- Unchecked exceptions. Also called runtime exceptions, these exceptions occur during program execution. These exceptions are not checked at compile time, so the programmer is responsible for handling these exceptions. Unchecked exceptions do not give compilation errors. Examples of unchecked exceptions include NullPointerException and IllegalArgumentException.
Exception handling in Java vs. exception handling in C++
Although the try, throw and catch blocks are all the same in the Java and C++ programming languages, there are some basic differences in each language.
For example, C++ exception handling has a catch all block, which can catch different types of exceptions, but Java does not. Likewise, C++ is able to throw primitives and pointers as exceptions, but Java can only throw objects as exceptions.
Unlike C++, Java has both checked and unchecked exceptions. Java also has a finally clause, which executes after the try-catch block for cleanup. C++ does not have a finally block. However, the finalize method will be removed in future versions of Java, which means users will have to find different methods to handle Java errors and cleanup.
Examples of exception handling
The following are examples of exceptions:
- SQLException is a checked exception that occurs while executing queries on a database for Structured Query Language syntax.
- ClassNotFoundException is a checked exception that occurs when the required class is not found -- either due to a command-line error, a missing CLASS file or an issue with the classpath.
- IllegalStateException is an unchecked exception that occurs when an environment's state does not match the operation being executed.
- IllegalArgumentException is an unchecked exception that occurs when an incorrect argument is passed to a method.
- NullPointerException is an unchecked exception that occurs when a user tries to access an object using a reference variable that is null or empty.
In this example, a variable is left undefined, so console.log generates an exception. The try bracket is used to contain the code that encounters the exception, so the application does not crash. The catch block is skipped if the code works. But, if an exception occurs, then the error is caught, and the catch block is executed.
Learn about the best practices behind exception handling for secure code design, including the process of throwing and handling different exceptions.