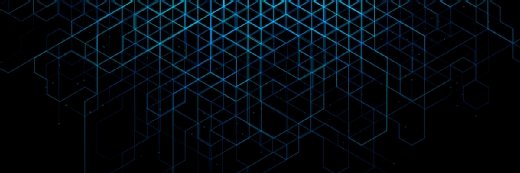
Getty Images
Breaking down the cornerstone components of OOP
Object-oriented programming changed the game for those working on complex software systems. But what are the key elements that define this prominent approach to development?
Object-oriented languages, such as Java, have formulated the ground rules for how developers generally handle data in complex software and web applications. More specifically, the object-oriented approach offers a development model that enables programmers to handle and organize that data as objects rather than blocks of code and logic.
At its core, object-oriented programming (OOP) allows developers to bind and manipulate data using exclusive functions. These functions cover a range of operations, including code reuse and variable designation. Programmers can then establish procedural code that governs data accessibility and makes it easy to add new functionality as applications and software architectures evolve over time.
In this article, we'll go over the essential components of OOP that define this approach to software development, including the four major principles it embodies, and explore how these concepts are embodied in programming languages like Java.
Objects, methods and classes
Adopting object-orientated programming starts with learning to clearly identify objects and define their relationships through data modeling and class designations. This requires developers to understand the following three main components of OOP: objects, methods and classes.
Objects
Collections of data and procedures are grouped into single, reusable entities. Objects feature two major characteristics: data, which describes an object's state and its unique characteristics, and behavior, which indicates how it interacts with other objects.
Class templates act as blueprints for creating individual objects. Once an object is instantiated from a class, developers define the object state using data in the object's attributes field while methods are also encapsulated within the object. Encapsulation exposes only selected information while hiding code inside a class and data within objects.
For example, OOP principles within Java allow programmers to differentiate objects from one another. They can then use data abstractions to hide details as needed and to design interfaces.
Methods
Developers invoke methods to manipulate data, promote reusability and encapsulate functionality inside an object. Defined within the class definition, methods deliver information about an object, update an object's data or delete and modify data as directed.
Along with encapsulating functionality, developers can create public methods and attach documentation so that other external developers can call methods on that object.
Classes
These define the state, behavior and identity of every object. Similar to a blueprint, a class organizes broad categories and defines the attributes that a certain object will have. Classes contain definable fields for designating attributes. Each class offers developers a template definition of the methods and variables contained in a specific object. Once developers define the variables and methods that can be referenced, they can better determine those properties associated with an object or shared among a group of objects.
Let's look at an example of how these components interact. In Java, class designation instructs a JVM environment to build an object. Using a single class definition, a programmer can build multiple objects into a software program. This component of OOP allows for faster parallel development, modular class reuse and a higher degree of maintainability.
The 4 major principles of OOP
The object-oriented approach is better defined as a set of guiding concepts than as a technical method. Specifically, there are four overarching OOP principles: inheritance, polymorphism, reusability and encapsulation.
Inheritance
Developers can simultaneously reuse common logic among unique objects, but only if they assign hierarchic relationships and subclasses to those objects. Programmers can use inheritance to avoid code duplication, simplify object design complexity or derive a new class from an existing class. For example, a child class inherits methods and fields of the parent class, which allows access to the same functionality as that parent class.
If a particular class can inherit fields and methods from a superior class, programmers can better manage the integration of all the objects involved. Class inheritance moves from the top of the hierarchy to the bottom, with the top made up of superclasses, which are also called parent classes. A superclass can maintain any number of subclasses, but a single subclass should never associate with more than one superclass. Inheritance not only supports the reusability of code to eliminate boilerplate rewriting, but it also helps to decrease the overall volume of code.
Polymorphism
Polymorphism means that objects can adopt more than one form, depending on their context. The application should then be able to independently recognize the correct form, execute the code and run the object's associated methods. This creates clear boundaries between entities that share the same name with signatures and declarations. It is the key OOP mechanism that allows components to share behavior.
Polymorphism helps in the dynamic calling of correct functions. For example, in a strictly typed language developers use polymorphism to assemble lists, collections or arrays of objects of different types and ensure that they comprise objects of the correct type. This not only makes processing the list of items more straightforward, it allows programmers to easily switch implementations.
For example, databases can be implemented in many ways, such as talking to a file system or an RDBMS server like MySQL. Using polymorphism, developers can define an interface that's most suitable and seamless to execute the correct database calls.
It's worth noting that polymorphism takes two unique forms in Java coding:
- Compile time polymorphism, where objects can share the same static name, despite embodying different parameters.
- Runtime polymorphism, which enables a subclass (or child class) to activate a method provided by one of its parent classes at runtime.
Reusability
When developers want to create a new class, but an existing class already contains the desired code, they can derive a new class from the old one. In OOP, these modular classes enable developers to reuse those classes in other applications and projects. This reusability opens the door for programmers to perform fixed sets of operations on particular objects and add functionality as the application and codebase evolves.
Reducing code repetition through reusability also helps developers know where to go if they want to adopt a common piece of functionality. Beyond single codebase creation, code reusability enables new frameworks and technologies that the software community adopts. For example, popular open source frameworks like React, generates voluminous documentation and libraries. An individual programmer can make reusable code available so that other developers can deploy that specific code within their own software builds.
Encapsulation
Encapsulation represents the mechanism for code to bind with the data it manipulates. This provides a shield that protects data from being accessed by any external code existing outside this shield. When that data is hidden, only a declared function within that class can access the variables or data. This will prevent unauthorized objects, as well as contain failures that would otherwise spread to other application components.
In OOP, it's generally considered bad practice to retrieve information from inside an object and write separate code to then perform the action outside of the object. The strength of encapsulation means that the functionality is defined only in one place, where the data is kept. Developers don't need to know details about the object's internals to use it effectively -- they only need to know the result produced by that method.