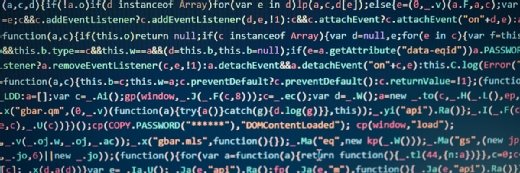
Getty Images
Test frameworks and examples for unit testing Python code
Unit testing is a crucial aspect of software development. Teams can adopt Python for unit testing to optimize Python's advantages and built-in framework options.
Unit testing allows QA testers and developers to eliminate problems in the codebase and elevate software quality. Choosing the right language and framework for unit testing is crucial for teams.
Unit tests are code-based tests that verify that a single component of code returns the correct results. They are typically automated and run during each code check-in or build. Unit testing is an important part of regression testing as it provides added assurance that bug fixes or defects from minor or major changes won't break existing functionality. Executing unit tests gives the software development team the ability to test all code in the code base, isolate errors, find hidden defects and reduce defect investigation time.
Python offers an open source option for unit testing that's concise and usable by a large variety of developer, DevOps and QA tester experience levels. Additionally, Python's built-in unit test frameworks can greatly benefit unit testing practices. Let's examine the advantages of unit testing in Python, some framework options and ways to combat common issues that may arise during unit testing.
Python frameworks and their unit testing advantages
Python is free, easy to set up and simple to use. More advantages of using Python over other languages for unit testing include the following:
- Compatibility with most tools and other coding languages.
- Object-oriented programming.
- Larger standard libraries compared to other languages.
- Built-in data structures.
- Automatic memory management.
- General-purpose language allows for common usage among developers with varying experience while also allowing for high-level programming.
Python's understandable and concise nature allows users to build more advanced unit tests in less time and with less test maintenance or debugging issues.
Another major advantage to using Python for unit testing is its abundance of built-in frameworks. Python has three built-in frameworks teams can use for unit testing: PyUnit, pytest and doctest. Developers who use one of these frameworks assist in creating on-point and simple unit tests that focus on verifying a single code component.
PyUnit is Python's built-in unit testing framework. PyUnit is like JUnit for Java. Start PyUnit by first importing the unittest library in Python. Unit tests created in PyUnit are subclasses of the unittest.TestCase class. Testers can then use the runTest() method to execute the unit tests with various assert functions.
Pytest is the alternative framework to the built-in PyUnit module that can be installed within Python. In pytest, developers write tests by writing functions prefixed with the word "test" and then letting pytest discover the tests automatically. The pytest framework uses the Python assert keyword. Once you write the test, save the file and then add both a setup and teardown. The pytest framework determines where fixtures are functions that have a return value. With pytest, the framework allows users to share fixtures across classes, modules, packages and sessions where fixtures can call other fixtures as arguments.
Doctest is another built-in test framework within Python. The primary purpose of doctest is to create unit test documentation. The doctest framework searches for code fragments that resemble interactive sessions and runs those sessions to verify they execute as expected.
Other tools available to support unit testing with Python include the following:
- Nose2. This is an extension to the unittest module. The nose2 tool provides an improved API plugin and more simplified internal interfaces and processes. It aids in test parameterization and fixture organization into layers, and allows for capturing log messages and provides test coverage data.
- Testify. This tool simplifies test assertion writing, mocks objects within the test frameworks and enables asserting on equal, not equal or nil.
Common unit testing problems
After choosing and implementing a testing framework, unit testing can often come with a variety of preventable problems. Teams can meet to discuss a strategy that avoids unnecessary delays and re-work.
Common unit testing problems include the following:
Developing the wrong types of tests. For example, say developers write system tests rather than testing a single component. System tests involve significant complexity based on application functions and integration points. While they are useful, they're not as beneficial as unit tests. Instead, developers should stick to simple, single-component tests for efficient unit testing suites. Simple tests are more readily debugged and require less maintenance time to keep them functioning.
Misunderstanding test doubles. This refers to coding mistakes when not understanding the function of a mock or stub. Mocks and stubs allow test development around connection points such as APIs and databases. When not done with a full understanding of the application functionality, the unit tests fail frequently. If mocks and stubs are used in a simple and understandable method, unit test suites benefit.
Frequent manual debugging. Frequent manual debugging means the unit tests have failed to automatically connect to the debugging system when a problem occurs.
Unclear test names. Unit test naming conventions need to include the function, intent or test objective. Using the test objective in the name of the test makes identifying any problems easier and creates easy test documentation of what code includes unit tests.
Skipping test maintenance. Test maintenance is efficient when unit test issues are noticed and immediately corrected. When test issues are ignored, or the test is not executed because a test script fails, then unit testing regression is incomplete and less useful. Correct failing or inaccurate tests and keep the entire unit test suite running as expected.