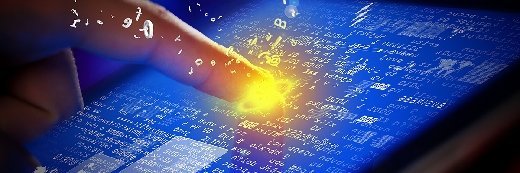
Sergey Nivens - Fotolia
Implement automated employee onboarding with PowerShell
Your time is precious and you shouldn't waste it by clicking through menus to set up a new user. Look at these code examples to put together your own provisioning script.
One of those most common tasks help desk technicians and system administrators handle is provisioning all the resources to onboard new employees.
Depending on the organization, these tasks may include creating Active Directory user accounts, creating home folders, provisioning new Office 365 mailboxes and setting up a VoIP extension in the phone system. With a little PowerShell coding, you can put together an automated employee onboarding script that does a majority of this work in little to no time.
To automate this process, it's essential to define all the tasks. Many companies have a document that outlines the steps to onboard a new employee, such as the following:
- create an Active Directory user account;
- create a user folder on a file share; and
- assign a mobile device.
When building a PowerShell script, start by researching if the basics required for automation are available. For example, does the system you're using to assign a mobile device have an API? If not, then it can't be completely automated. Rather than bypass this step, you can still add something to your script that sends an email to the appropriate person to complete the setup.
For other tasks that PowerShell can automate, you can start by scaffolding out some code in your script editor.
Build the framework for the script
Start by adding some essential functions to encapsulate each task. The following code is an example of build functions for each of the tasks we want to automate.
param(
[Parameter()]
[ValidateNotNullOrEmpty()]
[string]$CsvFilePath
)
function New-CompanyAdUser {
[CmdletBinding()]
param
(
)
}
function New-CompanyUserFolder {
[CmdletBinding()]
param
(
)
}
function Register-CompanyMobileDevice {
[CmdletBinding()]
param
(
)
}
function Read-Employee {
[CmdletBinding()]
param
(
)
}
This isn't our final code. This is just a brainstorming exercise.
Add the code to receive input
Notice the param block at the top and the Read-Employee function. This function receives any type of input, such as a CSV file or database. When we create a function, it's easy to modify the code if the method changes.
For now, we are using a CSV file to make the Read-Employee script below. By default, this function takes the CSV file path when the script runs.
function Read-Employee {
[CmdletBinding()]
param
(
[Parameter()]
[ValidateNotNullOrEmpty()]
[string]$CsvFilePath = $CsvFilePath
)
Import-Csv -Path $CsvFilePath
}
Add a Read-Employee reference below this function.
We have a CSV file from human resources that looks like this:
FirstName,LastName,Department
Adam,Bertram,Accounting
Joe,Jones,HR
We'll give the script a name New-Employee.ps1 with the CsvFilePath parameter.
./New-Employee.ps1 -CsvFilePath './Employees.csv'
Developing the functions
Next, fill in the other functions. This is just an example but should give you a good idea of how you could build your code for the specifics your automated employee onboarding script should have. For more information on the creation of the New-CompanyAdUser function, you can find more information at this blog post.
param(
[Parameter()]
[ValidateNotNullOrEmpty()]
[string]$CsvFilePath
)
function New-CompanyAdUser {
[CmdletBinding()]
param
(
[Parameter(Mandatory)]
[ValidateNotNullOrEmpty()]
[pscustomobject]$EmployeeRecord
)
## Generate a random password
$password = [System.Web.Security.Membership]::GeneratePassword((Get-Random -Minimum 20 -Maximum 32), 3)
$secPw = ConvertTo-SecureString -String $password -AsPlainText -Force
## Generate a first initial/last name username
$userName = "$($EmployeeRecord.FirstName.Substring(0,1))$($EmployeeRecord.LastName))"
## Create the user
$NewUserParameters = @{
GivenName = $EmployeeRecord.FirstName
Surname = $EmployeeRecord.LastName
Name = $userName
AccountPassword = $secPw
}
New-AdUser @NewUserParameters
## Add the user to the department group
Add-AdGroupMember -Identity $EmployeeRecord.Department -Members $userName
}
function New-CompanyUserFolder {
[CmdletBinding()]
param
(
[Parameter(Mandatory)]
[ValidateNotNullOrEmpty()]
[pscustomobject]$EmployeeRecord
)
$fileServer = 'FS1'
$null = New-Item -Path "\\\\$fileServer\\Users\\$($EmployeeRecord.FirstName)$($EmployeeRecord.LastName)" -ItemType Directory
}
function Register-CompanyMobileDevice {
[CmdletBinding()]
param
(
[Parameter(Mandatory)]
[ValidateNotNullOrEmpty()]
[pscustomobject]$EmployeeRecord
)
## Send an email for now. If we ever can automate this, we'll do it here.
$sendMailParams = @{
'From' = '[email protected]'
'To' = '[email protected]'
'Subject' = 'A new mobile device needs to be registered'
'Body' = "Employee: $($EmployeeRecord.FirstName) $($EmployeeRecord.LastName)"
'SMTPServer' = 'smtpserver.something.local'
'SMTPPort' = '587'
}
Send-MailMessage @sendMailParams
}
function Read-Employee {
[CmdletBinding()]
param
(
[Parameter()]
[ValidateNotNullOrEmpty()]
[string]$CsvFilePath = $CsvFilePath
)
Import-Csv -Path $CsvFilePath
}
Read-Employee
Calling the functions
Once you build the functions, pass each of the employee records returned from Read-Employee to each function, as shown below.
$functions = 'New-CompanyAdUser','New-CompanyUserFolder','Register-CompanyMobileDevice'
foreach ($employee in (Read-Employee)) {
foreach ($function in $functions) {
& $function -EmployeeRecord $employee
}
}
By standardizing the function parameters to have a single parameter EmployeeRecord which coincides to a row in the CSV file, you can define the functions you want to call in an array and loop over each of them.
Click here to download the code used in this article on GitHub.