event handler
What is an event handler?
In programming, an event handler is a callback routine that operates asynchronously once an event takes place. It dictates the action that follows the event. The programmer writes a code for this action to take place.
What is an event?
In programming parlance, an event is some action that indicates a user interacting with a program. For example, an event can be a mouse click or pressing a key on the keyboard. An event can be a single element or even an HTML document.
An event is a meaningful element of application information from an underlying development framework. There are many types of events, either from a graphical user interface (GUI) toolkit or some input-type routine.
On the GUI side, events include keystrokes, mouse activity, action selections or timer expirations. On the input side, events include opening or closing files and data streams, reading data and so forth.
The point is that when an event happens, something should happen in response to it. Code must be created to ensure that every event is understood, and the appropriate action fired in response.
How events and event handlers work
The simplest way to think of an event is as a signal. This signal is fired every time a user interacts with a program. When this happens, the operating system or browser must be notified of the resultant changes and the program must respond appropriately. Here's where an event handler -- specifically, event handler code -- comes in. The actions succeeding the event can be programmed using JavaScript code. This is embedded in the HTML code of the website.
Consider HTML as an example. When a user clicks on an HTML button, it is considered an event. Similarly, when HTML webpage loading has finished, it too is an event. If JavaScript is used on that HTML site, it will react to those events via event handlers. Event handler code will verify user input and actions as well as browser actions so the appropriate response occurs every time a page loads, when a page is closed, when a user clicks a button (also known as a click event), when a user inputs data into a form, and so on.
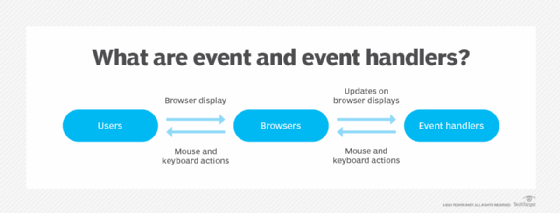
Here are some other common examples of an event handler:
- A notification pops up on a webpage when a new tab is opened.
- A form is submitted when the submit button is clicked.
- The background color changes on a mouse click.
Typically, these web events form a part of the browsers' API.
How to program and register an event in JavaScript
The general syntax for a handler is: <TAG eventHandler="JavaScript Code">
There are three common ways to handle events and register event handlers:
Method 1
Today, the standard way of registering an event handler is using the addEventListener() method. This method will register the handler as a listener for the element. The programmer enables this by specifying the event's name and the function that responds to it. Using this method, the handlers can also be removed using the method removeEventListener, making it the easiest way to handle events.
The syntax is: element.addEventListener("event name" , callback , useCapture)
Method 2
The second way to register an event handler is to use event objects as parameters. DOM elements contain methods (events) that can be used for event handling, such as the following:
- onclick. When a user clicks on an HTML element.
- onkeydown. When a user pushes a keyboard key.
- onchange. When an HTML element changes.
- onload. When the page finishes loading in the browser.
When a function is passed to these events, the event object is taken as a parameter.
The syntax for this approach is: element.onclick = (event)=>{…}
Method 3
A third type of event handler is to use HTML inline attributes. These attributes accept function calls as values and the event handlers return an event object as the callback parameter. This parameter contains the details about the event.
The syntax for this method is: <startTag onclick="myFunction()">content</endTag>
This method is not recommended for multiple reasons. One, inline HTML attributes inflate the markup and increase the mix of HTML and JavaScript code in the codebase, making it less readable and harder to understand. Additionally, adding too many inline attributes makes it difficult to debug the code and improve its quality. Inline event handlers in a single file can also increase the maintenance burden. To prevent these issues (and as a security measure), many server configurations disallow inline JavaScript.
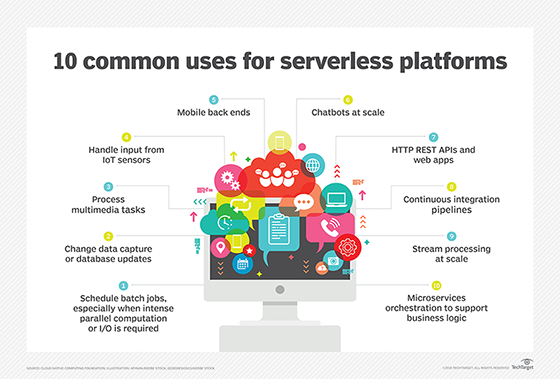
Registering event handlers with onevent properties
Here is a fourth method to register event handlers. The event handler code is assigned to the target element's corresponding onevent property. All JavaScript objects that fire events have a corresponding onevent property, which will be called to run the handler code when the event is fired.
With this method, only one handler can be assigned per event, unlike the event listener method where multiple event handlers can be added or removed. Also, by using an element's onevent property, the event handler can be replaced by assigning another function to that property.
The difference between event handlers and event listeners
The terms event handler and event listener are often used interchangeably. However, there is a slight difference between the two. These are two ways of handling events.
When a code runs after an event takes place, this is known as registering an event handler. On the other hand, the event listener listens to the event and then triggers the code for handling the event.
Event-driven software architectures
Nearly all software architectures include some event handling capabilities, if only to deal with out-of-bounds conditions and errors. Some software architectures operate almost entirely by producing, managing and consuming events.
These architectures are known as event-driven architectures and are common in modern microservices-based, cloud-native applications. Such applications rely on events to enable communications between decoupled services, which can be either event producers or event consumers. The producer publishes an event to an event router, which then pushes it to the appropriate consumer. Only when the event presents itself to the router, some action is taken.
Due to such on-demand action, event-driven architectures are "push-based." These architectures consume less network bandwidth, utilize less CPU and require fewer SSL/TLS handshakes. For these reasons, they are preferred for developing modern applications.
Learn how to handle typical event-driven architecture failures.