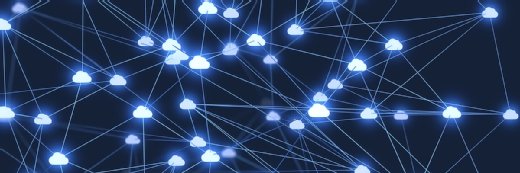
Getty Images
How to launch an EC2 instance using Terraform
With Terraform, developers can lean on familiar coding practices to provision the underlying resources for their applications. Follow these steps to use the IaC tool to create an Amazon EC2 instance.
Developers can create an Amazon EC2 instance to test software in a development or staging environment or to deploy software to production. With Terraform, they can use code to streamline that process.
An EC2 instance is a virtual machine that runs in the AWS cloud. AWS manages the underlying hardware, which lets users focus on the software they're running rather than infrastructure management tasks. Terraform is an infrastructure as code (IaC) tool that lets IT admins and developers programmatically provision infrastructure resources.
In this tutorial, learn about the benefits of Terraform and how to use it to launch an EC2 instance in AWS.
Why choose Terraform as a developer
Developers need to use their time wisely. As a result, they aim to minimize repetitive and manual processes.
Typically, developers focus on designing and planning application architecture as well as writing code. IaC tools like Terraform, which uses the HashiCorp Configuration Language, let developers apply familiar skills, such as writing code, to deploy the cloud infrastructure on which their software will run on. Terraform also lets developers write tests, including unit and integration tests; store those tests in source control; and collaborate on them with others.
Create an EC2 instance with Terraform
In this section, we'll write the code to create an EC2 instance. We'll review how to set up the main.tf file to create an EC2 instance and the variable files to ensure the instance is repeatable across any environment.
The following are the prerequisites for this process:
- AWS account.
- AWS Identify and Access Management credentials and programmatic access.
- AWS credentials set up locally with aws configure in the AWS Command Line Interface.
- A virtual private cloud (VPC) configured for EC2.
- A code or text editor.
For the purposes of this section, we will use Visual Studio Code as a code editor. However, any text editor will work.
Step 1. Create the main.tf file
Open your text/code editor and create a new directory. Make a file named main.tf. When setting up the main.tf file, you will create and use the Terraform AWS provider -- a plugin that lets Terraform communicate with the AWS platform -- and the EC2 instance.
First, add the provider code to ensure you use the AWS provider.
terraform {
required_providers {
aws = {
source = "hashicorp/aws"
}
}
}
Next, set up your Terraform resource, which describes an infrastructure object, for the EC2 instance. This will create the instance. Define the instance type and configure the network.
resource "aws_instance" "" {
ami = var.ami
instance_type = var.instance_type
network_interface {
network_interface_id = var.network_interface_id
device_index = 0
}
credit_specification {
cpu_credits = "unlimited"
}
}
Step 2. Create the variables.tf file
Once the main.tf file is created, set up the necessary variables. These variables let you pass in values and ensure the code is repeatable. With variables, you can use your code within any EC2 environment.
When setting up the variables.tf file, include the following three variables:
- network_interface_id. The network interface ID to attach to the EC2 instance from the VPC.
- ami. The Amazon Machine Image (AMI) of an instance. In the code snippet below, the AMI defaults to Ubuntu.
- instance_type. The size of the instance. In the code snippet below, the instance type defaults to a t2 Micro instance size.
Within the variables.tf file, create the following variables.
variable "network_interface_id" {
type = string
default = "network_id_from_aws"
}
variable "ami" {
type = string
default = "ami-005e54dee72cc1d00"
}
variable "instance_type" {
type = string
default = "t2.micro"
}
Step 3. Create the EC2 environment
To deploy the EC2 environment, ensure you're in the Terraform module/directory in which you write the Terraform code, and run the following commands:
- terraform init. Initializes the environment and pulls down the AWS provider.
- terraform plan. Creates an execution plan for the environment and confirm no bugs are found.
- terraform apply --auto-approve. Creates and automatically approves the environment.
Step 4. Clean up the environment
To destroy all Terraform environments, ensure that you're in the Terraform module/directory that you used to create the EC2 instance and run terraform destroy.
Editor's note: This article was republished to improve the reader experience.
Michael Levan is a cloud enthusiast, DevOps pro and HashiCorp Ambassador. He speaks internationally, writes blogs, publishes books, and creates online courses on various IT topics. He makes real-world, project focused content to coach engineers on how to create quality work.