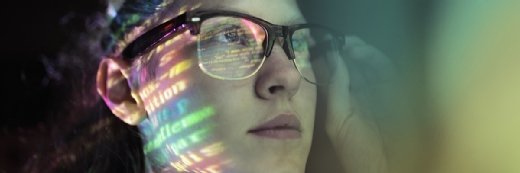
Getty Images
The basics of working with declarative programming languages
While imperative programming is often a go-to, the declarative approach has proved useful in the face of demands for complex, feature-heavy business applications.
When high-level programming languages first emerged in the 1950s, code was mainly based on combinations of command-based instructions and mathematical expressions. Because of this, imperative programming soon became a cornerstone approach to software development. However, over time, developers working on complex, feature-heavy application projects started to find the command-based nature of imperative coding painfully limiting.
One of the ways the development community tried addressing this issue was to create easy-to-read syntax that bore a closer resemblance to plain English, such as is found in languages like COBOL and BASIC. However, this also spurred increased adoption of the declarative programming styles found in languages like Prolog, Miranda and Haskell. While these languages were often associated with high-level computer science pursuits, such as compiler construction and database navigation, many web developers came to favor the declarative approach, noting its increased syntax legibility and reduced code footprint.
In this article, we'll take a deeper look into declarative programming languages, including the foundational aspects that make them appealing to business application and web developers. We'll also review examples of the different types of declarative programming approaches, and lay out the pros, cons and other factors that should influence a developer's decision to pursue the declarative paradigm.
What is declarative programming?
Declarative programming is a style of coding where developers express computation logic without the need to program the control flow of individual processes. This can help simplify coding processes, as developers need only describe what the program must achieve, rather than explicitly dictate the steps or commands required to achieve the desired outcome.
Put simply, declarative coding only instructs a program on what should be done, not how to do it. Of course, this means that it is critical to precisely and accurately specify the desired outcomes. However, instilling a focus on defining overarching goals, rather than manually calibrating each process, arguably reduces the chance that simple coding errors will cause an application to fail.
Many languages fall under the banner of declarative programming, but some of the most widely used include HTML, SQL, CSS and XML. Other examples include a mix of functional and logic programming languages, such as Prolog, Haskell, Miranda, XQuery and Lisp.
Pros and cons of declarative programming
Declarative programming languages often empower developers working on large, feature-heavy applications to improve productivity and code efficiency. The function-based structure found within declarative languages usually eliminates the need to write and maintain hundreds of lines of instructional code.
In addition to that, some of the other advantages associated with declarative programming are as follows:
- Declarative code is easy to read because developers declare what they want the program to do rather than describe how to do so.
- The declarative approach minimizes data mutability, which improves program security because immutable data structures are, in many cases, less prone to error.
- Declarative functions are typically reusable and sharable, but developers still retain the flexibility to implement new methods when needed.
Of course, the declarative approach presents its own set of challenges. Here are some of the most notable obstacles that developers confront:
- Declarative programming requires a certain degree of familiarity with abstract theoretical programming models, which not all developers have.
- Despite the potential for reusability, individual developers who want to personalize that sharable code may need to perform significant rewrites.
- In the event of an error, the ambiguity of control flow within the code can make it difficult to determine what specific actions an application performed without close examination of detailed application logs.
Declarative vs. imperative programming
In contrast to declarative programming, imperative programming requires developers to provide step-by-step commands that dictate the program's individual actions. Declarative programming encourages developers to focus on what the program ultimately needs to do; imperative programming requires developers to focus on exactly how the program will achieve its goal, one step at a time.
Imperative programming, of course, has some notable advantages of its own. For one, developers can manipulate certain instructions to directly change the state of an application when needed. Further, imperative programming lends itself to mutable states and loops that can execute multiple commands simultaneously. Finally, many developers already have some experience with imperative, as some of the most prominent languages today use this approach, including C, C++, Java and PHP.
One of the most standout differences between declarative and imperative is the actual amount of code there is to work with and maintain. The two code examples below are for a program that displays the last names of each person on a subscription list. The first takes an imperative approach, while the second takes a declarative approach.
In the imperative approach, the developer creates step-by-step instructions that tell the program to list each last name in order:
$participantlist = [1 => ‘Grey’, 2 => ‘Devon’, 3 => ‘Kor’];
$lastnames = [];
Foreach ($participantlist as $id => $name) {
$lastnames[] = $name;
}
In the declarative approach, the developer simply codes a function that is preconfigured to pull the names of participants and list those values in order:
$lastnames = array_values ($participantlist);
Styles of declarative programming
While declarative programming represents a unique spin on development, it also is an umbrella term for a variety of other programming styles. For instance, declarative programming can be divided into three subsets of paradigms: logic, functional and data-driven.
Logic programming
Though it is frequently described as an abstract computation model, logic programming is a coding style where the program's behavior is based on expressions of formal logic, typically in the form of mathematical statements. In this approach, the application uses a preexisting knowledge base to answer questions or perform certain actions.
A classic example of logic programming is Euclid's common notion. A logic programming statement could be as simple as the following:
- A is equal to B.
- B is equal to C.
- Therefore, A is equal to C.
Functional programming
The functional programming approach creates computations that represent specific application behaviors. Developers can adjust specific application functions and values without changing the underlying logic of the program. Often discussed as an alternative to object-oriented programming, this approach gained popularity with the rise of web development languages like JavaScript.
As an example, let's use JavaScript to identify if a given number is prime. Notice how the program uses mathematical calculations to determine its course of action:
function isPrime(number){
for(let i=2; i<=Math.floor(Math.sqrt(number)); i++){
if(number % i == 0 ){
return false;
}
}
return true;
}
isPrime(15); //returns false
Data-driven programming
As the name implies, data-driven programming focuses on data and its movement within a software system. For instance, developers can use a data-driven programming language such as SQL to access, modify and update data that resides within a large database. This approach is often used in business scenarios for tasks like file creation, data entry, information queries and reporting.
The example below shows the code for a program that pulls a data file containing detailed information about an individual on a customer list. Notice how this approach creates tables that process the necessary data:
CREATE DATABASE personalDetails;
CREATE TABLE Persons (
PersonID int,
Name varchar(255),
Email varchar(255),
Address varchar(255),
City varchar(255)
);
Should you use declarative programming?
Declarative programming languages can greatly reduce the management complexity of certain development projects but can also add complexity to applications that aren't feature-heavy or likely to grow in scale.
The process of establishing the outcome of a program, rather than commanding each step, provides an efficient and productive option for developers that work with particularly complex programs. At the same time, developers working on relatively straightforward applications that are unlikely to change in scale may find that imperative programming is the ideal approach.
Both imperative and declarative paradigms play critical roles in today's development scene; the choice of one or the other comes down to the precise details and requirements of a given project.