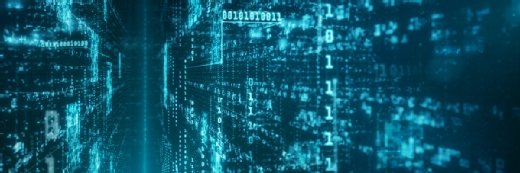
Getty Images
The basics of working with pseudocode
Writing pseudocode is a great way to practice problem-solving skills, a crucial aspect in programming. It helps developers conceptualize and communicate their ideas.
Transforming an idea into a functional program involves a series of structured steps. Before the code is written and the program is tested, there's a critical phase where the logic and structure of the program are conceptualized. This is where pseudocode comes into play.
As a vital bridge between the conceptualization of a program and its implementation in a programming language, pseudocode enables developers to articulate their ideas clearly and concisely.
Understanding how pseudocode fits into the overall development process, along with its principles, best practices and use cases, can help programmers optimize their approach and proactively address errors or potential oversights that could lead to future complications.
What is pseudocode?
Pseudocode is a readable description of a program or algorithm that uses everyday language rather than a specific programming language. It serves as a high-level design tool, enabling developers to conceptualize algorithms and processes without getting bogged down in syntax. Compared to flow charts and other algorithm-designing tools, pseudocode's simplicity and accessibility make it a popular choice among developers.
At its core, pseudocode is a way to express the logic of a program or algorithm without the constraints of a specific programming language's syntax. Unlike actual program code, it's not meant to be compiled or run. Instead, pseudocode helps the programmer think through a problem and devise a plan for solving it. It enables easy modifications and is a quick way to write ideas and algorithms.
Advantages of using pseudocode
Using pseudocode in the development process offers assorted benefits, including the following:
- Improved clarity. Provides a simplified algorithm representation, enabling problems and solutions to be more easily understood.
- More accessible communication. Facilitates the clear communication of ideas, fosters collaboration and ensures that everyone involved understands the algorithm.
- Streamlined debugging. Saves time and resources by identifying logical errors or inefficiencies before the code-writing process.
- Universality. Offers an accessible medium for developers proficient in various programming languages to understand and work together on a project, especially as pseudocode does not depend on any particular language.
- Educational tool. Enables learners to focus on logic rather than syntax and is helpful for teaching programming concepts.
- Efficient planning. Reduces errors by organizing program structure before diving into the coding.
Getting started with pseudocode
To write effective pseudocode, follow these basic principles:
- Use everyday language. Write in simple terms, making it easy for others to understand the algorithm.
- Include essential details. Clearly describe the algorithm's inputs, outputs and necessary steps.
- Keep it concise. Avoid long or complex explanations.
First, understand and analyze the problem that needs solving. Next, break down the problem and identify its smaller, more manageable parts. Then, start writing pseudocode for each part, clearly describing the necessary steps for solving them. Lastly, integrate the individual pseudocode sections into a cohesive whole.
Pseudocode examples
Here are a couple of pseudocode examples illustrating the application of these principles in practice. The first example depicts a binary search algorithm for locating a target item in a sorted array. The second example outlines a bubble sorting algorithm for organizing items in a specific sequence.
Binary search
FUNCTION binarySearch(array, target)
left = 0
right = array length – 1
WHILE left <= right
middle = (left + right) / 2
IF array[middle] == target
RETURN middle
ELSE IF array[middle] < target
left = middle + 1
ELSE
right = middle – 1
END IF
END WHILE
RETURN 'Not Found'
END FUNCTION
Bubble sort
FOR i = 1 to array length – 1
FOR j = 1 to array length – i
IF array[j] > array[j + 1]
SWAP array[j] and array[j + 1]
END IF
END FOR
END FOR
Best practices for writing pseudocode
Pseudocode can make the coding process smoother and more efficient. Here are some tips for writing effective pseudocode:
- Define the problem clearly. Before writing pseudocode, thoroughly understand the problem. Outline the desired outcomes, inputs and necessary steps to reach the outcome.
- Keep it simple. Use straightforward and concise language that accurately depicts the steps of the algorithm. Avoid using complex terms or jargon that might confuse others.
- Use consistent language. To maintain clarity, use a consistent style throughout the pseudocode. This helps others better understand the thought process behind the approach.
- Write in a logical order. Each step should follow a logical sequence. Translating pseudocode into a programming language is easier that way.
- Review and refine. Upon completion, ensure the pseudocode is clear and logical. Look for areas that could use simplification or clarification.
Pseudocode doesn't have to be perfect. Its main purpose is to help conceptualize the algorithm and facilitate communication with others.
Pseudocode in different programming paradigms
Pseudocode is adaptable, helping to bridge the gap between high-level ideas and specific programming constructs for different programming paradigms, including the following two:
- Procedural programming. In procedural programming, code is written as a sequence of instructions. Pseudocode can outline the procedures and the order in which they should be executed. For instance, a programmer could use pseudocode to write out the steps to calculate the factorial of a number before implementing it in a procedural language.
- Object-oriented programming (OOP). OOP focuses on objects and their interactions. Pseudocode in OOP might outline the classes, their properties and methods. For example, one could use pseudocode to design a car class with properties (color and speed) and methods (accelerate and brake) before implementing it in an OOP language, such as Java.
In both paradigms, pseudocode enables developers to focus on the logic and structure of the program without getting entangled in the syntax. Practicing pseudocode across different paradigms can strengthen an individual's understanding of core programming concepts and improve their versatility as a developer.
Where pseudocode fits best
Pseudocode is a powerful tool for programmers. It offers a simplified way to design, communicate and debug algorithms. For seasoned developers, pseudocode can help with the design of complex algorithms and systems. It enables developers to express their ideas clearly, collaborate effectively with their peers and catch potential errors early in development.
For beginners, pseudocode is an invaluable tool for learning programming fundamentals. It provides a way to focus on understanding the logic and structure of an algorithm without the added complexity of syntax.
Twain Taylor is a technical writer, musician and runner. Having started his career at Google in its early days, today, Twain is an accomplished tech influencer. He works closely with startups and journals in the cloud-native space.