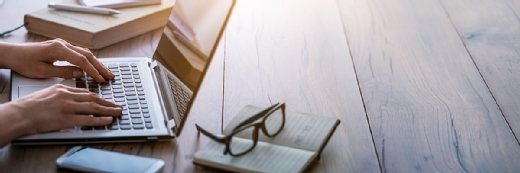
Getty Images/iStockphoto
How to script a Bash shell argument
This tutorial teaches you how to add arguments to your Bash scripts, which can simplify and automate a variety of Linux tasks in the data center and eliminate hassle.
Linux shell scripts make it possible for admins to create tasks and automate them with the help of a tool such as cron. Shell scripts can be as simple as a single line command, or can contain multiple nested lines that run numerous tasks.
Shell scripts are often called Bash scripts because Bash is the most common default shell in Linux. They call upon one or more applications to handle various jobs. You can use Bash scripts to pass arguments to those internal applications, which means you don't have to edit the script when values related to those applications change.
For example, if you use AppX in a shell script and AppX requires you to let it know that DIRECTORYZ is the location that houses the necessary data to complete its task, you could write a line in the shell script reads something like:
/usr/bin/AppX -d /DIRECTORYZ
When you no longer want AppX to use DIRECTORYZ, or if AppX must use a dataset from different directories depending on the situation, you can either manually edit that line in the script to reflect the correct dataset or you can employ arguments.
What are arguments?
Arguments represent different options passed to a command. You can add the following to the example shell script to create more arguments:
ls -I README file 'file 1' file2
The command now has five arguments:
- /usr/bin/ls, the actual command,
- -I, instructing ls to ignore the next string,
- file, a file to be listed,
- file 1, the next file to be listed,
- and file2, the final file to be listed.
The same command also has three positional parameters:
- file
- file 1
- file2
A script designates positional parameters as $0, $1, $2, $3, $4 and so forth. These parameters enable you to pass arguments from the command line to a script.
Crafting a script to use arguments
Every Bash script begins with the line:
#!/bin/bash
That tells the script to use Bash. A simple script enables you to input arguments that are passed to the script and then used for output.
The first section of the script prints out the total number of arguments to pass to the scripts and then prints out the value of each argument. That section looks like this:
echo "Total Number of Arguments:" $#
echo "Argument values:" $@
$# expands to reflect the number of arguments passed to a script. This means you can enter as few or as many arguments as you require. When you use this variable, it automatically designates the first variable at $0, the second at $1, the third at $2 and so on.
The second variable, $@, expands to all parameters when calling a function. Instead of listing the total arguments as a value, it lists them all out as typed.
To create the actual Bash script, issue the command:
nano script.sh
Into that script paste the following:
#!/bin/bash
echo "Total Number of Arguments:" $#
echo "Argument values:" $@
Save and close the file. Give the file executable permission with the command:
chmod u+x script.sh
If you run the script without passing arguments, the output is:
Total Arguments: 0
All Arguments values:
Instead, run the script and pass arguments with the command:
./script.sh ARG0 ARG1 ARG2 ARG3 ARG4 ARG5 ARG6 ARG7 ARG8 ARG9
The output of that command is then:
Total Arguments: 10
All Arguments values: ARG0 ARG1 ARG2 ARG3 ARG4 ARG5 ARG6 ARG7 ARG8 ARG9
You can make that same script more useful by accessing only specific arguments. For example, if you want to print out the first and last argument, you could include:
echo "First Argument:" $1
echo "Tenth Argument:" ${!#}
The script now uses $1 for the first variable, because $0 prints out the command ./script.sh as its first argument. The {!#} variable, the last argument passed to the script, combines the argument count with indirection, which enables you to reference something using a name, reference or container instead of the value itself.
Add the second section to the script, so it looks like:
#!/bin/bash
echo "Total Number of Arguments:" $#
echo "Argument values:" $@
echo "First Argument:" $1
echo "Last Argument:" ${!#}
If you run that command like so:
./script.sh ARG0 ARG1 ARG2 ARG3 ARG4 ARG5 ARG6 ARG7 ARG8 ARG9
The output should be:
Total Arguments: 10
All Arguments values: ARG0 ARG1 ARG2 ARG3 ARG4 ARG5 ARG6 ARG7 ARG8 ARG9
First Argument: ARG0
Last Argument: ARG9
Simplifying the Bash scripting process
You can make this script a bit more practical. For example, you could create a backup script that uses rsync to back up a directory to a USB-attached drive mounted at the /backup directory.
If you previously backed up the same directory, that script might look like:
#!/bin/bash
rsync -av --delete /Directory1/ /backup
Use arguments to define the directory to be backed up from the terminal. The new script looks like:
#!/bin/bash
rsync -av --delete $1 /backup
Call that script backup.sh.
If you have a directory -- for the purposes of this example, a directory named PROJECT4 -- you can back it up to the /backup directory by issuing the command:
./backup.sh PROJECT4
You can then back up any directory you want by passing the name of the directory to the backup script as an argument.
You can build on these fundamental exercises to expand your knowledge of arguments. Bash scripts can become incredibly powerful tools to simplify Linux administration tasks.