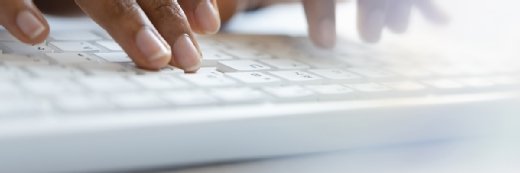
Getty Images
Try these PowerShell Start-Job examples for more efficiency
It might take some effort to rework scripts to take advantage of PowerShell jobs capabilities, but it will free you to handle other work while running code behind the scenes.
Hurry up and wait. If you're not working with jobs in PowerShell, then this might sound painfully familiar.
Many administrators who use PowerShell will eventually develop advanced scripts to handle complex tasks. While much of this automation work runs fairly quickly, there will be situations when a script requires a significant period of time to complete. Staring at the screen as you wait for a script to finish is not the most efficient use of time. One option is to use PowerShell jobs, which is a valuable way to run several scripts in parallel without tying up the console until they complete.
How can job parallelization help? I can attest to its value through personal experience. I have a script that would take more than an hour to pull in data before it finished a complicated task that involved several infrastructure components. After I reworked the code to use PowerShell jobs, the script took half the time to run with the added benefit of freeing the PowerShell console to run other commands.
What is a PowerShell background job?
The Start-Job cmdlet begins a background job for the current PowerShell session and runs the code asynchronously. That script will run, and you can continue to use PowerShell to handle other tasks. A PowerShell job is ideal for work that does not require user input or for automated tasks that run on a schedule.
PowerShell assigns an ID to the background job. You can use the ID to check on the current status of the job and get results from any data collected by the code.
What modifications will your scripts need?
Converting your PowerShell code to run as a background job may require some retooling and effort. A requirement to be aware of with PowerShell jobs is there is no way for interactive input. The background state makes the job inaccessible from the console.
When a PowerShell job runs, there is no native error reporting; the script will fail silently if any problems occur. Therefore, your code will need scrutiny and testing to avoid any snags that could prevent it from clean execution.
One way to tidy up a script is to export details or checkpoints at key parts of the script to a text file. This checks the script's activity to assist troubleshooting if an error occurs. This can be as simple as exporting a useful note such as "pre-processing complete" or "API query complete" and recording the variables. It depends on the level of information required.
How to work with PowerShell jobs
Three main cmdlets manage jobs in PowerShell: Start-Job, Get-Job and Stop-Job. To run a job is quite straightforward:
Start-Job -ScriptBlock {}
The PowerShell system runs the code in the ScriptBlock parameter. A simple example is:
Start-Job -ScriptBlock { Get-Content c:\mybigdatafile.txt }
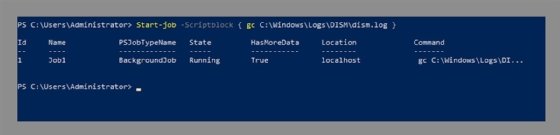
It is also possible to directly run a script in the background with the following PowerShell Start-Job example:
Start-Job -FilePath c:\code\myscript.ps1
The script runs but cannot accept input from the console.
When running several jobs in the background, you can check the status of these jobs with the Get-Job cmdlet.
As the name suggests, Get-Job shows the running jobs and the status of each. Even if you submit several jobs, they are not sequential because every Start-Job creates a child process to do the work.
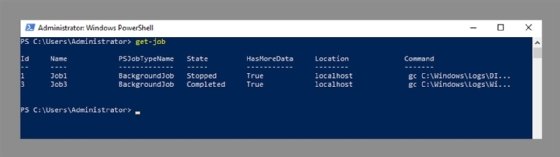
The output of the Get-Job command shows the script status. This is invaluable when you need to know the status of a script running in the background.
Sometimes a job may go wrong or need to be stopped. The Stop-Job cmdlet will halt a script using the following format:
Stop-Job <JOB ID>
For additional details to work with these cmdlets, PowerShell's built-in help using the Get-Help command provides an abundance of documentation and command examples.
Try these strategies to rework your code
How does one develop code to use parallel processing? First, make sure the code doesn't require interaction. You can write the script to gather data, then execute the jobs with the Start-Job cmdlet.
All the required inputs must be provided in advance with variables or however you gather the data, such as an API call that needs credentials for access.
Break down the code into functions. This makes it easier to manage and integrate into jobs. One way to get the job output is to use the transcript function. This is a quick way to record output from the script without needing to build your own reporting.
Using the PowerShell Start-Job cmdlet makes the script and you much more efficient. There are caveats with code that needs to be self-sufficient, but it is worth the time to understand how to add this flexibility to your scripts. The job management system works on most platforms that will run PowerShell, such as Linux and macOS, as well as Windows.