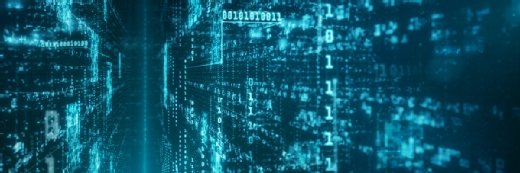
Getty Images
How to use PowerShell with the Azure REST API
With PowerShell automation coupled with the Azure REST API, it's easy to build a script to create, power on and remove virtual machines in Microsoft's cloud platform.
There are many ways to interact with Azure, but PowerShell might be the most familiar for many administrators who work in a Windows environment.
IT workers who need to work with users in Azure Active Directory, virtual machines and other Azure resources can interact with the cloud platform directly with Azure cmdlets. But there are times when it is simpler -- or required -- to use the Azure REST API.
For example, if there was a requirement to incorporate the work into a web application, then the API would be the most efficient way to integrate with Azure. While this article looks at using the Azure REST API with PowerShell, it's just one example of the method and would work with some slight adjustments using another language.
For the sake of brevity, this article will only cover how to build a request, not the authorization aspect of Azure. Using the API to create a virtual machine rather than cmdlets means breaking down the task into several components that make up the request.
How to build the Azure REST API request in PowerShell
Azure PowerShell uses the Invoke-RestMethod cmdlet to use JSON to communicate with Azure.
The following is a typical API request performed using PowerShell:
$result = Invoke-RestMethod -Method Post -Body $MyData -ContentType "application/json" -Authorization $headerdata
The Method parameter tells the endpoint what type of operation to perform with the request. To send data, choose the Post value in most scenarios.
The Post value, as the name implies, pushes data into the API environment while the Get parameter pulls data out of the environment.
The Body parameter is where the data is pushed to Azure. Creating a VM with an API call means passing a lot of configuration data in the body of the request into Azure for processing.
Trying to pipe all that data inline into the request is unwieldy and could cause errors. It makes sense to keep all the required body information, specifically the VM data, in an external file and pull it into a variable. This makes for a script that is tidy and easier to maintain. It also makes it simple to have several files with different configurations, such as different Azure regions.
Be sure to import all values, meaning those that come from a file, as raw JSON; otherwise, PowerShell will import it into a new PowerShell object and it can't be used for direct API work.
Before running the script, populate the $MyData variable to include the above code before the API call.
$MyData = Get-Content myvmdata.json -Raw | ConvertFrom-Json
This code primes the data variable to be created in Azure via an API request.
Users with some familiarity with PowerShell can use Invoke-WebRequest to pass the data. An example of the data required to create a VM JSON file can be found at Microsoft's documentation site here.
When submitting a request, make sure to substitute your own resource group and subscription ID into the request. This information is available from the Azure portal and via API requests.
PUT https://management.azure.com/subscriptions/{subscription-id}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}?api-version=2017-12-01
After it is created, the VM appears as another VM in Azure. To work with the VM, there are several API requests available to make adjustments, such as turning off the VM, changing networks and changing disks.
Using PowerShell and the Azure REST API to adjust VMs
Administrators will want to do more than just create VMs; they will want to delete them, power them off and power them on.
The code below demonstrates how to use Post to push the command into the Azure environment to power off the VM.
The URL data is configured in $MyData. Users need to adjust the resource data, but notice how when we issue the request in the $result portion of the request, it is identical to the one we used before. The implementation data comes from the $MyData variable.
$MyData="https://management.azure.com/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/powerOff?api-version=2020-06-01" $result = Invoke-RestMethod -Method Post -Body $MyData -ContentType "application/json" -Authorization $headerdata
Repurpose to code and use the same call and change the $MyData as follows to start the VM.
$MyData="https://management.azure.com/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/start?api-version=2020-06-01"
It's simple and straightforward to interact with Azure to create and manage your VM resources with PowerShell and the Azure REST API. It is not limited to any single language and the examples mentioned only require slight adjustments to port them over.
More details about how to work with Azure VM APIs can be found here.