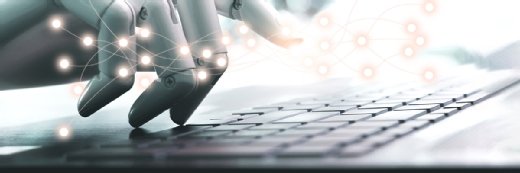
Getty Images/iStockphoto
How to use GitHub Copilot for PowerShell coding projects
The integration of this code completion tool with VS Code can benefit administrators who want to automate jobs. But there are some limitations with this fledgling technology.
For IT admins who code with PowerShell, the future is now.
Generative AI is finding its way into every aspect of our lives, including the tools and technologies used by IT personnel. GitHub Copilot uses AI to assist users in their coding efforts. This tool, used in tandem with Visual Studio Code (VS Code), can help admins climb the PowerShell learning curve by producing code based on prompts.
How GitHub Copilot works
In some quarters, AI is a controversial technology. It requires an incredible amount of processing power to review and process existing content, then uses algorithms to produce works of its own through user prompts. In the case of GitHub Copilot, it scans public GitHub repositories to understand how to write code and then applies its findings to help other users produce scripts.
If you are new to PowerShell, then you stand to learn a lot from GitHub Copilot and will be able to produce impressive code in a short amount of time. However, because GitHub Copilot bases its expertise on humans who make mistakes, there is a chance the results might not be ideal or may use outdated information.
How to prepare to use GitHub Copilot
You need a GitHub account to sign up for GitHub Copilot.
Once you sign in, navigate to the Settings section and then the Copilot page. You can start with the 60-day free trial. After that, GitHub Copilot costs $10 a month or $100 a year for an individual and $19 per user for the Copilot for Business plan.
During the signup process, you have the choice to get suggestions that match public code and whether you want to submit your own code snippets for product improvements. These are both optional.
Next, you install the GitHub Copilot extension and approve the authorization for VS Code when prompted. The Copilot icon should appear in the bottom-right corner of the VS Code window.
How to write PowerShell code with GitHub Copilot
Even with an AI pair programming tool, you cannot produce code without giving the product a starting point. At its most basic, what do you want the PowerShell script to accomplish? GitHub Copilot needs direction from you to understand how it should proceed. One way to begin is to give Copilot a description of what the script should do. A little PowerShell understanding will also help you steer GitHub Copilot to give you the right code.
For this example, let's say you want to see all the running Windows services on a machine. As an admin who works with Windows systems, you want to see the logon account for each service.
First, write the following comment in VS Code and hit Enter.
# Get all running services
For me, GitHub Copilot produced the following code.
Get-Service | Where-Object {$_Status -eq "Running"}
To accept the GitHub Copilot suggestion, press Tab.
Next we want to assign that PowerShell command to a variable. This will likely require a foreach loop, so enter the following comment.
# foreach service, get the account the service is running as
Press Enter to see the code suggestion from GitHub Copilot.
# foreach service, get the account the service is running as
foreach ($service in $services) {
$serviceAccount = Get-WmiObject -Class Win32_Service -Filter "Name='$($service.Name)'" | Select-Object -ExpandProperty StartName
Write-Host $service.Name $serviceAccount
}
The result is impressive, but there is a problem. The code works for Windows PowerShell 5.1, but I use PowerShell 7, which doesn't have the Windows Management Instrumentation cmdlets. I adjusted the comment to indicate I needed compatible code for PowerShell 7, which GitHub Copilot then provided.
# foreach service, get the account the service is running as in PowerShell 7
foreach ($service in $services) {
$serviceAccount = Get-Service -Name $service.Name | Select-Object -ExpandProperty UserName
Write-Host $service.Name $serviceAccount
}
As advanced as GitHub Copilot is, changing your comment might not work for every command. GitHub Copilot suggested that we pipe the output to Select-Object and reference the UserName property.
The comment we entered for a prompt would be better if we used our PowerShell understanding to reference the UserName property. By adjusting the comment, we get different code from GitHub Copilot.
# foreach service, output the username
foreach ($service in $services) {
$service | Select-Object Name, DisplayName, Status, UserName
}
These AI coding tools are still not a substitute for someone with a sufficient level of PowerShell expertise. You might get code that works, but it might not be efficient. For example, in the previous code, the first foreach look calls the Get-Service cmdlet every time, which is unnecessary because the code already has this information stored in the $services variable.
How to script an Azure VM deployment with GitHub Copilot
GitHub Copilot automates many server-related jobs, such as a simple PowerShell script that deploys a new VM in Azure.
First, start with a descriptive comment.
# Azure VM deployment script
After pressing Enter twice, GitHub Copilot suggested the following.
# Login to Azure
This makes sense, because we need to sign in to Azure to access our subscription and its cloud resources. Pressing Tab completed the suggestion, but GitHub Copilot suggested using Login-AzureRmAccount, which is outdated, rather than the Connect-AzAccount cmdlet in the newer Az PowerShell module.
However, after manually typing out the correct command and hitting Enter a couple of times, GitHub Copilot adapted and offered to select a subscription with the proper cmdlet, even suggesting the comment to keep the style the same throughout.
# Set the subscription
Set-AzContext -SubscriptionId "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
GitHub Copilot then recommended a resource group.
# Create a resource group
New-AzResourceGroup -Name "RESOURCE_GROUP_NAME" -Location "LOCATION"
GitHub Copilot continued with coding for a virtual network, a subnet, a public IP and a network interface.
# Create a virtual network
New-AzVirtualNetwork -ResourceGroupName "RESOURCE_GROUP_NAME" -Name "VNET_NAME" -AddressPrefix ""
# Create a subnet
Add-AzVirtualNetworkSubnetConfig -Name "SUBNET_NAME" -AddressPrefix "" -VirtualNetwork $vnet# Create a public IP address
New-AzPublicIpAddress -ResourceGroupName "RESOURCE_GROUP_NAME" -Name "PUBLIC_IP_NAME" -AllocationMethod Dynamic -DomainNameLabel "DOMAIN_NAME_LABEL"
# Create a network interface
New-AzNetworkInterface -ResourceGroupName "RESOURCE_GROUP_NAME" -Name "NIC_NAME" -Location "LOCATION" -SubnetId $subnet.Id -PublicIpAddressId $publicIp.Id
Next, GitHub Copilot produced the PowerShell code to build, start and get the Azure VM.
# Create a virtual machine
New-AzVM -ResourceGroupName "RESOURCE_GROUP_NAME" -Name "VM_NAME" -Location "LOCATION" -VirtualNetworkName "VNET_NAME" -SubnetName "SUBNET_NAME" -SecurityGroupName "SECURITY_GROUP_NAME" -PublicIpAddressName "PUBLIC_IP_NAME" -OpenPorts 80,3389 -VirtualNetwork $vnet -NetworkInterfaceName "NIC_NAME" -Image "IMAGE_NAME" -Size "SIZE_NAME"
# Start the virtual machine
Start-AzVM -ResourceGroupName "RESOURCE_GROUP_NAME" -Name "VM_NAME"
# Get the virtual machine
$vm = Get-AzVM -ResourceGroupName "RESOURCE_GROUP_NAME" -Name "VM_NAME"
If you continue, then GitHub Copilot will propose to include the other supporting resources such as a storage account.
For this project, I wrote a minimal amount of code. Aside from correcting the login command, I just had to fill out the parameter names and assign output to variables. Other than testing the script, my work was done. GitHub CoPilot saved me about an hour or more of work to write a script based on the prompts I provided.
GitHub Copilot will not solve every problem and will not always write perfect PowerShell code. But it can help you update existing scripts and develop new ones. It can be particularly helpful when you are under a deadline and need to produce an automation script as fast as possible.