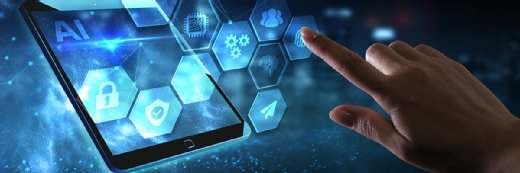
putilov_denis - stock.adobe.com
How to use ChatGPT with PowerShell to write code for admins
Use of artificial intelligence tools is on the rise in many industries, and administrators can tap into these digital helpers to improve their scripting attempts.
Interest in artificial intelligence and how it can ease our lives has surged, including in the IT field.
With the proliferation of humanlike chatbots and generative AI that produces various media, AI is seemingly everywhere. Whenever there is a technology that can replicate what a human does, there is a tendency to be fearful and wonder whether jobs are in jeopardy. But AI can be a boon particularly for admins who want to create scripts in PowerShell and improve the ones they currently use. One offering that is particularly promising is OpenAI's ChatGPT, which can write PowerShell code, provide coding ideas and even troubleshoot a faulty script.
Let's take a closer look at what ChatGPT is, how much it costs, how to use it with PowerShell and what to watch out for.
What is ChatGPT?
ChatGPT is OpenAI's natural language model AI that you access through a web-based chat interface. You type in prompts, and the AI responds, much like an online conversation. It's an astonishingly simple and accessible AI.
The free version uses OpenAI's GPT-3.5 model. Subscribers who pay $20 a month get ChatGPT Plus with more advanced capabilities and can access both the GPT-3.5 model and the GPT-4 model. Open AI said the paid tier offers faster response, access at peak times, and browsing and plugin access.
OpenAI also offers a product called ChatGPT Enterprise, which does not have a public pricing model, but it reportedly has improvements in coding and handles larger inputs.
If you plan to use ChatGPT heavily or in a professional setting, the upgrade to ChatGPT Plus might be worth it. For casual users, it might be better to stay with the free tier.
If you are integrating ChatGPT or any other AI from OpenAI, you can also use its API's usage-based model with pricing that depends on the AI module. All examples in this article reference the web-based chat interface.
To start, open an account with OpenAI on the login page for ChatGPT.
What are the benefits of using generative AI for PowerShell scripting?
One advantage of PowerShell scripting with a natural language model AI, such as ChatGPT, is you tell the AI what you want and it creates compelling code. It's a more efficient way to write code compared to entering search terms in Google and piecing together the information yourself. Your interactions with ChatGPT are conversational, so you can guide the AI to the information you want by building on earlier responses.
For example, say you want to write a script to find all VMs in Azure with a specific tag and to shut them down. Before AI, your process might be to examine the output of the Get-AzVM PowerShell command, look for a property with tags to filter on and then look at the syntax of the Stop-AzVM cmdlet. You can prompt ChatGPT to write the script:
Write a script that retrieves all virtual machines from Azure that have the tag 'shutdown' with the value 'daily' and then shuts those virtual machines down.
I didn't specify PowerShell, nor did I suggest any methods to complete the action. Here is the script that was generated:
# Connect to Azure
Connect-AzAccount
# Define the resource group and tag criteria
$resourceGroup = "YourResourceGroup"
$tagName = "shutdown"
$tagValue = "daily"
# Retrieve the virtual machines with the specified tag
$vms = Get-AzResource -ResourceGroupName $resourceGroup -Tag @{ $tagName = $tagValue } | Where-Object {$_.ResourceType -eq "Microsoft.Compute/virtualMachines"}
# Stop each virtual machine
foreach ($vm in $vms) {
$vmName = $vm.Name
Write-Host "Stopping virtual machine: $vmName"
Stop-AzVM -ResourceGroupName $resourceGroup -Name $vmName -Force
}
ChatGPT did not use the Get-AzVM cmdlet, which is what I expected. Rather, ChatGPT used the Get-AzResource cmdlet, which can filter left and only retrieve resources with the specified tag. I wasn't aware of this functionality, so I learned something new.
Another thing to highlight is how the script handles resource groups. The ChatGPT PowerShell script assumes the VMs are in the same resource group. However, each object that Get-AzResource outputs includes the name of the resource group. You could instead reference that value to retrieve the name of the resource group.
Knowing that, ask ChatGPT to fix it with the following prompt:
The output from 'Get-AzResource' includes the resource group name, could you use that with 'stop-azvm' instead of hardcoding the resource group name?
And it replies with a new version of the script:
# Connect to Azure
Connect-AzAccount
# Define the tag criteria
$tagName = "shutdown"
$tagValue = "daily"
# Retrieve the virtual machines with the specified tag
$vms = Get-AzResource -Tag @{ $tagName = $tagValue } | Where-Object {$_.ResourceType -eq "Microsoft.Compute/virtualMachines"}
# Stop each virtual machine
foreach ($vm in $vms) {
$vmName = $vm.Name
$resourceGroup = $vm.ResourceGroupName
Write-Host "Stopping virtual machine: $vmName"
Stop-AzVM -ResourceGroupName $resourceGroup -Name $vmName -Force
}
This script completes the task.
It is important to review the code carefully. In this case, the worst that happens is the script throws an error if you try to shut down a VM with the wrong resource group. However, we caught the error and made the correction because we knew enough PowerShell to identify the problem and ask ChatGPT to update it.
How to use ChatGPT to improve PowerShell code
Another benefit of using ChatGPT with PowerShell is to troubleshoot or improve existing code. For example, say you have the following script that needs to get all open TCP connections and link them to a running process:
Get-NetTCPConnection | foreach-Object {
[pscustomobject]@{
LocalAddress = $_.LocalAddress
LocalPort = $_.LocalPort
RemoteAddress = $_.RemoteAddress
RemotePort = $_.RemotePort
State = $_.State
OwningProcess = $_.OwningProcess
CreationTime = $_.CreationTime
PID = $_.OwningProcess
Process = (Get-Process -Id $_.OwningProcess).Name
UserName = (Get-Process -IncludeUserName -Id $_.OwningProcess).UserName
Path = (Get-Process -Id $_.OwningProcess).Path
SHA1 = try{(Get-FileHash (Get-Process -Id $_.OwningProcess).Path -Algorithm SHA1 | select-object -ExpandProperty Hash)}catch{};
CommandLine = (Get-Process -Id $_.OwningProcess).CommandLine
}
}
On my computer, the script takes around two minutes to run. You can prompt ChatGPT to optimize the PowerShell code to see if it runs faster:
Can you optimize this PowerShell script for me?
<paste in code here>
ChatGPT responded with the following updated PowerShell script:
$tcpConnections = Get-NetTCPConnection
$connectionInfo = foreach ($connection in $tcpConnections) {
$process = Get-Process -Id $connection.OwningProcess -ErrorAction SilentlyContinue
[pscustomobject]@{
LocalAddress = $connection.LocalAddress
LocalPort = $connection.LocalPort
RemoteAddress = $connection.RemoteAddress
RemotePort = $connection.RemotePort
State = $connection.State
OwningProcess = $connection.OwningProcess
CreationTime = $connection.CreationTime
PID = $connection.OwningProcess
Process = if ($process) { $process.Name } else { $null }
UserName = if ($process) { $process.UserName } else { $null }
Path = if ($process) { $process.Path } else { $null }
SHA1 = try { (Get-FileHash $process.Path -Algorithm SHA1).Hash } catch { $null }
CommandLine = if ($process) { $process.CommandLine } else { $null }
}
}
$connectionInfo
When I ran the code, it generated an error.
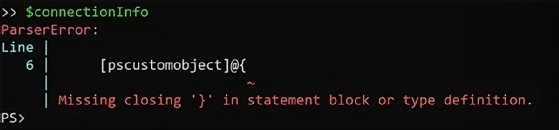
This is unfortunate because ChatGPT was not able to help. I pasted the error verbatim and asked ChatGPT to make the correction, but it repeatedly gave the same code until I explained the fix:
The SHA1 line, where you have the 'try catch' block, actually needs a semicolon at the end of the line for the code to work.
With my help, ChatGPT produced working code after it added the semicolon to line 18. The good news is the updated script takes 35 seconds instead of two minutes.
ChatGPT's optimization strategy for this script is good. The first version of the script repeatedly called the Get-Process cmdlet and often retrieved the same process by id. ChatGPT avoids this slowdown by querying the owning process once per loop.
ChatGPT can be helpful but there are caveats
As we've seen with the previous examples, ChatGPT is not perfect. With any code that it produces, you should carefully examine it and, especially if you don't understand it, run it cautiously. You should consider using the -WhatIf parameter to view changes before they occur or comment out any cmdlets that make the changes while you run tests.
Keep in mind that, as a user of ChatGPT, you are training it. Anything you use to prompt ChatGPT and any code you submit for examination should not be considered private. If you are writing proprietary code, then you may run afoul of your company's policies when you paste scripts into ChatGPT.
If you haven't tried ChatGPT yet, then you should. AI is here to stay, and ChatGPT is incredibly accessible and has a generous free tier. You will likely run into areas where ChatGPT doesn't understand or cannot produce the desired results on the first prompt, but you should keep trying. It requires a new skill to understand how to phrase requests in a different way or to consider a new approach to get the PowerShell code tailored to your task.