Spring Framework (Spring)
What is Spring Framework (Spring)?
Spring Framework (Spring) is an open source software development framework that provides infrastructure support for building Java-based applications on any deployment platform.
Released in June 2003 by Rod Johnson under the Apache 2.0 license, Spring Framework is hosted by SourceForge.
What is the Spring framework used for?
One of the most popular Java Enterprise Edition (Java EE) frameworks, Spring helps developers create high-performing applications using plain old Java objects (POJOs) and servlet containers that speed up development. In addition to POJOs, Spring uses techniques like aspect-oriented programming (AOP) and dependency injection to simplify development and reduce application testing and maintenance costs. These framework qualities make Spring highly suitable for building reliable and scalable enterprise applications in Java EE 7, while simplifying the development process.
In contrast to Spring, classic frameworks and application programming interfaces (APIs), like Java Database Connectivity (JDBC), JavaServer Pages and Java Servlet, can make building Java applications a more complex and time-consuming endeavor.
Apart from Spring and JavaServer Faces, other popular Java frameworks include Maven, Hibernate and Struts.
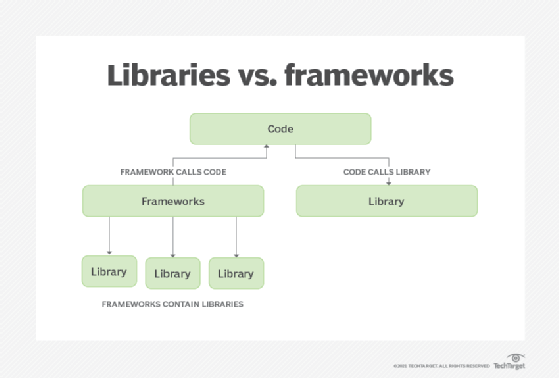
Why Spring Framework for Java applications?
Many Java programs are complex and feature many components that are dependent on the underlying operating system for their appearance and properties. Spring provides a way to simplify the complexity and easily resolve the various technical problems that developers may encounter.
Spring is widely considered to be a secure, low-cost and flexible framework that improves coding efficiency and reduces overall application development time through efficient use of system resources. Spring removes tedious configuration work so that developers can focus on writing business logic.
In addition, Spring handles the infrastructure so developers can focus on developing high-quality, useful and scalable enterprise applications without having to worry about deployment environments and other infrastructural concerns.
And Spring provides numerous loosely coupled modules or subframeworks, also known as layers, to improve the functionality of a web application. These modules, such as Spring AOP, Spring Object Relational Mapping, Spring Web Flow and Spring Web model-view-controller (MVC) may be used separately or together, providing greater flexibility during development, especially for developing applets and enterprise-class applications.
How Spring Framework works
A web application (layered architecture) commonly includes three major layers:
- A presentation layer, or user interface, that handles the display of content and user interactions.
- A business logic layer that deals with the underlying, functional specifications of a program.
- A data access layer that supervises data retrieval procedures.
Each layer is dependent on the other for an application to work. For instance, the presentation layer might need to talk with the business logic layer, which, in turn, interacts with the data access layer. Each of these interactions leads to what are commonly referred to as dependencies and creates couplings between various system components.
One of Spring's core traits is its ability to perform dependency injection, which is a programming pattern that enables developers to build more decoupled architectures. Spring understands different Java annotations that a developer puts on top of classes and can help make sure that all instances created have properly populated dependencies.
For Spring Framework to instantiate objects and populate the dependencies, a programmer simply tells Spring which objects to manage and what the dependencies are for each class through annotations. For instance, the @component annotation lets Spring know which classes to manage, marks objects as managed components and identifies classes for dependency injection. As another example, the @autowired annotation tells Spring how to handle the instantiation of the class and to start looking for that dependency among components and classes to find a match.
Important technologies and features of Spring
Spring Framework includes numerous technologies that differentiate it from -- and, in many cases, make it a better choice than -- other Java frameworks.
Inversion of control
Inversion of control (IoC) is a design principle in software engineering that gives control execution to the framework -- in this case, Spring. By taking control over the program's flow and making calls to custom code, Spring makes it easier to build modular programs, test programs and switch between different implementations as needed.
Dependency injection
Dependency injection, a specialized form of IoC in Spring, is a design pattern in which objects define their dependencies in one of three ways:
- Constructor arguments.
- Arguments to a factory method.
- Properties set on the object instance after it is constructed or returned from a factory method.
An assembler connects, or injects, objects with other objects. Dependency injection promotes loose coupling in code, so changes in one area of the application are less likely to affect another.
With dependency injection, the code is cleaner. Furthermore, IOC and dependency injection make it easier to test and maintain code.
IoC container
This is the portion of Spring Framework where objects are created, wired together, configured and managed throughout their lifecycle. Its main purpose is to instantiate, configure, assemble and manage Spring beans. The container reads the configuration metadata -- containing instructions from the developer and represented in Extensible Markup Language, Java annotations or Java code -- to understand which objects (beans) to instantiate, configure and assemble. Explicit user code is usually not required to instantiate one or more instances of a Spring IoC container.
Beans
In Spring Framework, beans are the objects that form the backbone of any application. Beans are managed by the Spring IoC container and represented in the IoC container as BeanDefinition objects. All beans and their dependencies are reflected in the configuration metadata used by a container and supplied by the application developer. Every bean has a unique identifier, although it can have other identifiers, known as aliases.
Other important terms in Spring
Here are a few other important terms associated with Spring that developers should know before getting started with the framework:
- Autowiring collaborators. This is the process by which Spring automatically resolves the collaborators (other beans) for a bean. Developers also have the option to switch to explicit wiring once the codebase stabilizes.
- Lazy-initialized beans. Bean definitions can be marked as lazy-initialized to tell the IoC container not to create a bean instance at startup, or preinstantiation, but when it is first requested.
- Singleton and nonsingleton beans. With a singleton bean, Spring scopes a single bean definition to a single object instance for each IoC container. Singleton beans can collaborate with other singleton beans if the developer handles the dependency by defining one bean as a property of the other.
- Bean scopes. Spring supports six bean scopes: singleton, prototype, request, session, application and websocket. Developers can define beans to deploy them in one of these six scopes. Spring also supports custom scopes, although it is not possible to override the built-in singleton and prototype scopes.
Advantages of Spring Framework
The key advantage of Spring is that it removes many of the complexities associated with Java programming and helps speed up application development and testing processes. This is because it is a lightweight framework, supports loosely coupled applications, and provides predefined templates for JDBC, Hibernate, etc. Another advantage is that Spring is a noninvasive framework since it doesn't force developers to inherit classes or implement interfaces during development.
With Spring, developers can easily write code to use persistence APIs to store and access persistence data in a database. They can also build web applications built on Spring Web MVC architecture, a web framework built on the Servlet API with support for diverse workflows.
Spring supports two languages: Kotlin and Groovy. Kotlin enables developers to write concise and elegant code and is interoperable with existing Java libraries. Groovy offers a concise syntax and smooth integration with existing Java applications. In addition, Spring supports the use of classes and objects defined with a supported dynamic language so developers can use Spring IoC containers to transparently instantiate, configure and dependency-inject the resulting objects.
Other benefits and advantages of Spring include the following:
- It integrates with numerous technologies, including representational state transfer clients, Java Management Extensions, Java Message Service and Java virtual machine Checkpoint Restore.
- The correct use of IoC simplifies both unit testing and integration testing.
- It provides comprehensive transaction management support with a consistent programming model, a simpler API for programmatic transaction management and integration with Spring's data access abstractions.
- With Spring Expression Language, object graphs can be queried and manipulated at runtime.
- Spring's AOP component, with aspects defining modularity, enables the modularization of concerns across multiple types and objects.
A good integration framework should underpin every application strategy and can simplify integration at scale. Explore top integration frameworks: Apache vs. Spring vs. Mule.