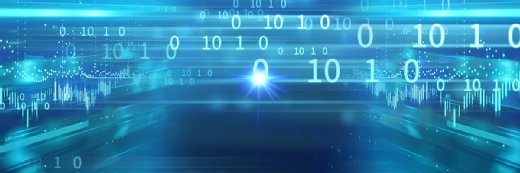
Getty Images/iStockphoto
Learn by doing with these PowerShell regex examples
When you must find specific text in a large file, such as a system log, regular expressions can come to the rescue. Learn how to add this skill to your administrative arsenal.
Understanding how to use regular expressions to match patterns in strings is a skill that can benefit every IT administrator.
The ability to define a pattern with text can help you in several ways, such as uncovering pertinent information from a lengthy log file. Regular expressions sift through data quickly, which helps manage and monitor Windows-based infrastructure. Regex can be used in different languages, but this tutorial will focus on showing several PowerShell regex examples to explain how to execute the searches.
What are regular expressions?
A regular expression, also known as regex, is a special sequence of characters used to match a pattern in a string. For example, you can build a regular expression to find a credit card number inside a string. You start by constructing a pattern to match the sequence of four groups that consist of four numbers separated by a space, such as 0123 4567 8901 2345.
In regular expressions, the \d sequence matches a single digit from 0 to 9. The following regular expression would match the example of a credit card number.
'\d\d\d\d \d\d\d\d \d\d\d\d \d\d\d\d'
The following PowerShell regex cheat sheet shows other special sequences in regular expressions used to find a match.
Sequence | Matches on |
\d | Single digit |
\D | Single character that is not a digit |
\s | Single whitespace character |
\S | Single character that is not whitespace |
\w | Single word character |
\W | Not word character |
\n | New line |
. | Anything but new line |
This PowerShell regex cheat sheet is useful for a beginner to learn the basics of pattern matching.
How to form PowerShell specific regular expressions
Programming languages use their own regular expression engines, meaning a regular expression that works in PowerShell might not work in Perl or Python. PowerShell uses the C#/.NET regular expression engine. If you use an online regular expression tester, then you should check if it is compatible with the scripting language you use.
PowerShell uses regular expressions two ways: in conditional statements using the -match operator and with the Select-String cmdlet. The -match operator is limited to one regular expression at a time while Select-String can search for multiple regular expressions and through multiple files at once. Select-String can highlight matches, while -match cannot. Another benefit of Select-String is it can store matches into a variable.
How to work with the PowerShell -match operator
You use conditional statements in PowerShell to determine a true or false evaluation. You can use a regular expression to check if a string holds a phone number. You use the same approach as the credit card number example by using a 3-3-4 format.
'\d\d\d-\d\d\d-\d\d\d\d'
Use the -match operator to compare a string to the regular expression.
'123-456-7890' -match '\d\d\d-\d\d\d-\d\d\d\d'
PowerShell's Boolean operation will return true because it found a match for the regular expression.
You can place a phone number inside a longer string to see if there is a match with the regular expression.
'Call me: 123-456-7890 ASAP' -match '\d\d\d-\d\d\d-\d\d\d\d'
This will also return a true condition because the -match operation seeks a matching regular expression anywhere in the string.
Every time you use the -match operator and it returns a true condition, then PowerShell also creates the $Matches variable. In the example, if you enter the $Matches variable, then PowerShell will display the phone number it matched.
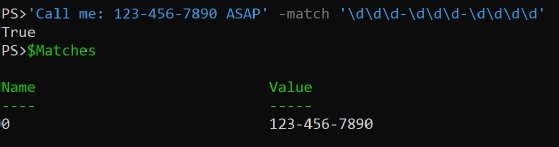
In the PowerShell output, the 0 label refers to the regular expression matching group. PowerShell creates a group when it finds a match. You can manipulate these labels for different purposes. For instance, if you want to create a separate group for the first three digits in a U.S. phone number, then you could create a group in a regular expression with the following syntax.
'Call me: 123-456-7890 ASAP' -match '(\d\d\d)-\d\d\d-\d\d\d\d'
Then, $Matches will show that group in its output.
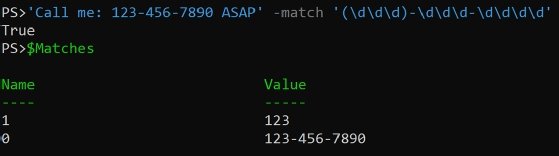
A handy feature of groups is name assignment. Sometimes grouping gets complex, making it difficult to know which group is assigned to which number. The following PowerShell regex example assigns the group a name inside angle brackets with the following syntax.
'Call me: 123-456-7890 ASAP' -match '(?<areaCode>\d\d\d)-\d\d\d-\d\d\d\d'
When you run the PowerShell -match operation again, then the areaCode group holds the match for the area code.
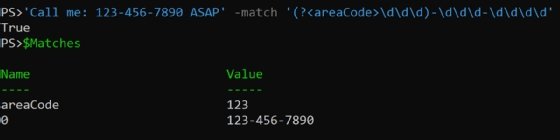
You can reference the areaCode value with the following code.
$Matches.areaCode
PowerShell will output the 123 value.
How to use regular expressions with Active Directory
Administrators in a Windows environment can use regular expressions for tasks related to infrastructure management. For example, it's not uncommon to find phone numbers in Active Directory user accounts in several formats:
- 123-456-7890
- 123 456 7890
- 1234567890
- (123) 456 7890
- (123) 456-7890
To account for all these scenarios, you can use ?, | and {} characters. The question mark is one of several PowerShell regular expression quantifiers, which dictate how many times a preceding character or group of characters should be matched. The question mark means a match of zero or one time. The pipe character acts as an "or" operator to match the regular expression pattern on the left side or the pattern on the right. The curly braces let you specify the quantity for the preceding character or group.
The PowerShell regular expression to match the different phone number formats looks like the following:
'\(?\d{3}\)?(-| )?\d{3}(-| )?\d{4}'
The backslash is the escape character, which means it dictates an exact match for the character that follows it. For example, to match a period you would use \. in the regular expression.
Let's break down the regular expression above to explain how it works:
- \(? indicates there could be an opening parenthesis or not.
- \d{3} indicates there should be three numbers.
- \)? indicates there could be a closing parenthesis or not.
- (-| )? Indicates there could be a dash or a space or not.
- \d{3} indicates there should be three numbers.
- (-| )? indicates there could be a dash or a space or not.
- \d{4} indicates there should be four numbers.
A more advanced technique is to place named groups around each of the sets of numbers.
'\(?(?<areaCode>\d{3})\)?(-| )?(?<first>\d{3})(-| )?(?<second>\d{4})'
When you -match a phone number, you can format it to your preference.
$regex = '\(?(?<areaCode>\d{3})\)?(-| )?(?<first>\d{3})(-| )?(?<second>\d{4})'
$phoneNumbers = @(
'123-456-7890',
'123 456 7890',
'1234567890',
'(123) 456 7890',
'(123) 456-7890'
)
foreach ($pn in $phoneNumbers) {
if ($pn -match $regex) {
"($($Matches.areaCode))-$($Matches.first)-$($Matches.second)"
}
}
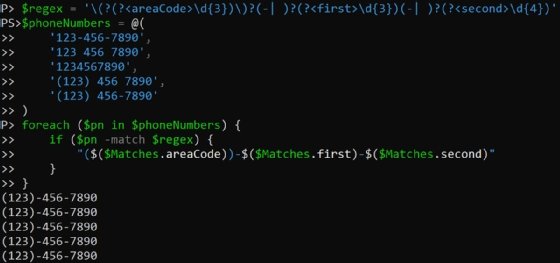
How to use the Select-String cmdlet for PowerShell regular expressions
The Select-String cmdlet is another option to use regular expressions in PowerShell. While it has some similarities with the -match operator, Select-String can work in the PowerShell pipeline against output from other cmdlets.
For example, to search the contents of files for a specific pattern, you can pipe the Get-Content command straight into the Select-String cmdlet. Working off the previous phone number example, you can look for phone numbers in a file by combining the two PowerShell commands.
Get-Content $filePath | Select-String -Pattern '\(?(?<areaCode>\d{3})\)?(-| )?(?<first>\d{3})(-| )?(?<second>\d{4})'
When you run this PowerShell code on a sample CSV file, then your output might look like the following screenshot.
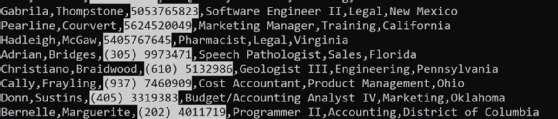
PowerShell highlighted the matching strings, which helps make specific text stand out when you must sift through a large amount of output data.
How to use regular expressions with Azure AD and Microsoft Graph
Another useful example for admins is to parse Get-Help output from PowerShell cmdlets to learn about their capabilities. For example, if you wanted to create a new user in Azure Active Directory with the Microsoft Graph module, then you'll find that the New-MgUser cmdlet has 130 parameters.
You can use Select-String to highlight all the parameters with the word Name with the following simple regular expression.
'-[^ ]+Name'
These characters in a regular expression will do the following:
- - looks for a dash.
- [^ ] specifies a character set but because the first character inside the square brackets is a caret, this negates the set. In this case, the regular expression will match on anything that is not a space.
- + qualifies the previous character to look for one or more of the preceding element, which is one or more characters except for spaces.
- Name is the literal string to find with the regular expression.
The following PowerShell command uses this regular expression with the Select-String cmdlet.
help New-MgUser | Select-String '-[^ ]+Name'
The PowerShell output makes it easy to find the parameters with Name.
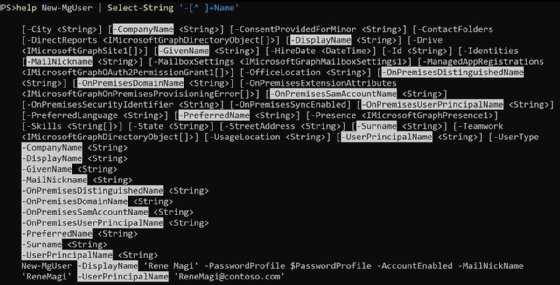
Find more help with basic PowerShell regular expressions in PowerShell
This is just the starting point to get a general understanding on how to build and use regular expressions in PowerShell. There are several online resources that help you work with regular expressions in PowerShell:
- Microsoft regular expression documentation.
- The Regex101 tester with the .NET (C#) setting.
- The Regex Generator.
- RegexOne tutorials.
- RegExHelper is a GUI tool written in PowerShell to help write regular expressions.
Use regular expressions in moderation
Knowing how to use regular expressions is a powerful skill, and it might be tempting to use them to parse everything. If you find yourself trying to parse a common data structure, such as HTML, always check for a parser and use that instead. This will let you finish the work quickly with better results so you can apply your time to more productive areas.