How to create a smart contract using Ethereum
Solidity is a powerful language for programming and deploying smart contracts on the Ethereum network. Here's how to get started, with advice on choosing the best tools.
Smart contracts, which are programs that run on a blockchain, have transformed blockchain from a data storage technology to an application platform that allows developers to support a variety of business processes, from supply chain management to real estate administration to decentralized finance.
At the center of it all is the Solidity programming language.
Although smart contracts can be written in a number of programming languages, Solidity has become the first choice for many developers doing smart contract programming. That's why it's worth knowing about.
Solidity is a powerful and complex programming language that takes time to master. However, starting out with Solidity isn't daunting, particularly for developers who have a background in object-oriented programming (OOP). This article shows you how to get started.
But before we get to the nuts and bolts of the matter, let's look at why Solidity has become so popular.
Smart contracts for the Ethereum network: How they work and why they're popular
One of the biggest reasons for Solidity's popularity is that it's the language used for programs that run on the Ethereum Virtual Machine (EVM). To understand why this is significant, it's useful to understand a bit of the history of blockchain.
Blockchain technology became commonplace with the introduction of the cryptocurrency Bitcoin in 2009. When it was first introduced, the Bitcoin blockchain supported transferring Bitcoin (the cryptocurrency) between parties. But, other than that, the Bitcoin blockchain was dumb. It didn't support executing rules or enforcing conditional logic. You couldn't program a transaction such as "have Bob transfer two Bitcoin to Alice on the first of the month as payment for rent on Bob's apartment."
However, another blockchain network came along that did have this sort of capability. That network was Ethereum. Solidity is the way Ethereum supports executing rules or enforcing conditional logic by way of smart contracts, and it is the language Ethereum created for writing smart contracts. Ethereum also specified the EVM as the mechanism for running smart contracts on the Ethereum blockchain.
The EVM acts as an intermediary between the smart contract's compiled bytecode and the Ethereum blockchain, very much in the same way that a Java virtual machine acts as the intermediary between compiled Java bytecode and the computer hosting the Java program.
The way smart contract development works under Ethereum is that developers write smart contract code in Solidity as a text file. Then, they use a tool called the Solidity compiler (solc) to transform the Solidity text into bytecode that the EVM can understand. Finally, the bytecode is deployed to and stored on the Ethereum blockchain at a particular address (see Figure 1.)
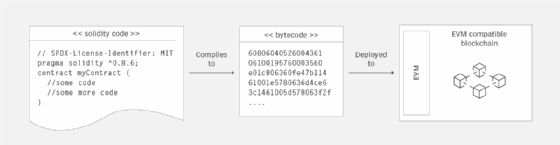
Depending on how it's programmed, the smart contract can run on its own continually, or other smart contracts can call functions in the smart contract by using special tools.
Solidity and the EVM caught on. Eventually, other blockchains such as Avalanche and Hedera incorporated the EVM specification. As support for the EVM grew among different blockchains, so too did the popularity of the Solidity programming language. Today GitHub reports over 56,000 source code repositories related to Solidity.
Creating and deploying a smart contract to the Ethereum network
Now, let's create a smart contract and show how to deploy it on Ethereum.
The Solidity programming language supports inheritance, encapsulation and polymorphism as is typically found in OOP languages such as Java, C# and TypeScript. Whereas in OOP the basic unit of encapsulation is the class, in Solidity it's called a contract. A contract can contain public or private data members and functions.
One added feature that is special to Solidity is the notion of the predefined msg, tx and block variables, which are global to any contract. The msg variable describes the external call made to the contract. The tx variable describes the fee associated with the cost of contract execution as well as the party that is going to pay that fee.
A feature common to all blockchains is that there is a fee, measured in a unit of denomination called gas, incurred every time a transaction is executed, regardless of whether the transaction is a simple transfer of funds or a function being executed in decentralized applications such as Solidity contracts.
The block variable describes the state of a block on the underlying blockchain on which the contract is hosted. For example, the property block.gaslimit reports the maximum amount of gas the underlying blockchain will allow to be consumed by a given transaction.
As with object-oriented programming, Solidity supports interfaces, thus allowing developers to define the methods and data members that an implementing contract will provide. Also, a smart contract is considered a composite data type just as class is in OOP.
The following code examples demonstrate the steps in creating a smart contract that declares an interface and then implements that interface in another Solidity smart contract. The final step shows yet another smart contract that returns the interface of the implementation contract.
Listing 1 declares an interface named ILocation. (All the code for this article is available in one of my GitHub repositories here.)
// SPDX-License-Identifier: MIT pragma solidity ^0.8.17; interface ILocation { function getLongitude( ) external view returns(uint16 ); function getLatitude( ) external view returns(uint16 ); }
Listing 2 shows how to implement the ILocation interface in a contract named Location. Notice that the syntax to declare a contract as an implementation of an interface is expressed at line 5 using the is keyword.
// SPDX-License-Identifier: MIT pragma solidity ^0.8.17; import "./ILocation.sol"; contract Location is ILocation { uint16 latitude; uint16 longitude; constructor (uint16 _longitude, uint16 _latitude){ latitude = _latitude; longitude = _longitude; } function getLongitude() external view returns(uint16 ){ return longitude; } function getLatitude () external view returns(uint16 ){ return latitude; } }
Listing 3 shows another Solidity smart contract that imports the Location contract defined in Listing 2. Notice the import statement at line 3. Importing the Location contract implicitly imports the ILocation interface too. The function getLocation() returns an ILocation interface which is backed behind the scenes by a Location contract defined in Listing 2.
// SPDX-License-Identifier: MIT pragma solidity ^0.8.17; import "./Location.sol"; contract LocationFactory { function getLocation(uint16 _longitude, uint16 _latitude) public returns (ILocation) { return new Location(_longitude, _latitude); } }
The three listings not only demonstrate how to create and use contracts in Solidity programming, the code also demonstrates how to use the program to the interface technique, which is common practice among object-oriented programmers.
When it comes time to deploy the code to an EVM-compatible blockchain, first the developer will compile the code into bytecode and then deploy the bytecode to the blockchain either using a tool at the command line or from within an IDE such as VS Code or Remix.
Let's look at the details.
Tools for writing a smart contract in Solidity
The evolution of tools available to create distributed applications based on smart contracts is impressive, given that Solidity was first proposed in 2014. Today, IDEs do most of the heavy lifting, allowing developers to focus on the logic of their smart contracts instead of the minutiae that goes with getting it all to work. Most developers use one for writing smart contracts, and the days of only using a simple text editor are long gone.
There are two types of IDEs. One type is hosted on a local machine, the other is online and accessed from a web browser. IDEs for local machines include VS Code and JetBrains WebStorm (see Figures 2 and 3).


Development in a local IDE will also involve using frameworks such as HardHat and Truffle to help execute compilation and deployment.
As for online tools, Ethereum's Remix is the most popular (see Figure 4).
Remix reduces the labor of creating Ethereum smart contracts by doing common development chores such as library management, code autocompletion, syntax checking and even deployment. Remix allows you to deploy smart contracts to development EVMs that are special to Remix. Also, you can bind the IDE to a MetaMask wallet browser plugin and then use test Ether cryptocurrency from a variety of Ethereum accounts to fund the transaction fees that go with deploying and running the smart contract to the blockchain.
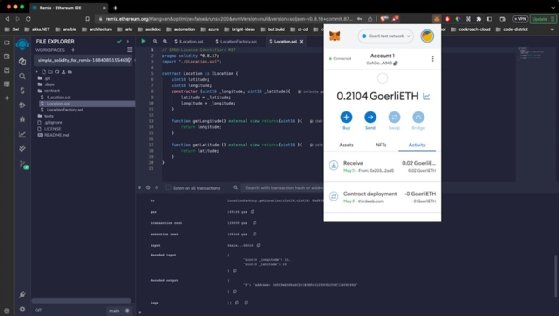
In conclusion
Solidity is a powerful language that blends the power of OOP with the intricacies of blockchain. A lot more Solidity programming opportunities will become available as Web 3.0 and decentralized applications continue to grow on the modern technical landscape. This article is but a first step on the exciting journey ahead.