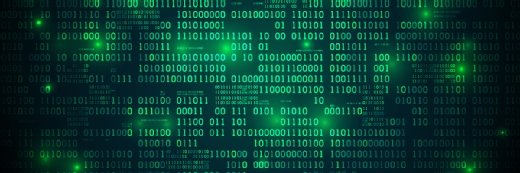
Getty Images/iStockphoto
Learn how to use the PowerShell Trim method
With the Trim method in your PowerShell toolbox, you can write scripts to remove unwanted characters from strings without using regular expressions. Get started with this tutorial.
All the work you do with PowerShell that deals with input or imported data depends on the quality of that data. When working with scripts that ingest data, you can use techniques such as parameter validation, regular expressions and even manual review. But sometimes you'll need to clean the data yourself.
In PowerShell, one tool for working with strings is the Trim() method, which removes unwanted characters from the start and ends of strings. In some cases, using Trim() even eliminates the need to write regular expressions, simplifying your code and creating a better experience for others who use your scripts.
Although all the examples in this tutorial are executed in PowerShell 7, they'll work the same in Windows PowerShell unless stated otherwise.
Call the Trim() method without parameters
In PowerShell, strings are considered a primitive type, meaning they're built into the language. Like any variable in PowerShell, strings have methods and properties.
Properties are static values associated with a variable, whereas methods perform a specific action when called. Unlike properties, methods are called with parentheses at the end, which sometimes contain parameters.
You can call the Trim() method without any parameters to remove spaces at the start and end of a string. For example, executing the Trim() method on a string variable $str with the value ' string ' outputs 'string', as shown in Figure 1.

You can test this yourself by running the following code, which shows that the output string is two characters shorter. Because it is difficult to display spaces in console output, this example uses the Length property, which returns the number of characters in a string.
$str = ' string '
$str
Write-Host "Length: $($str.Length)"
$str.Trim()
Write-Host "Trimmed Length: $($str.Trim().Length)"
Importantly, the Trim() method does not update the original variable; it simply returns the modified string to the console. To update the $str variable with the trimmed version of the string, run the command $str = $str.Trim(). As with all variable assignments, there is no output, but you can validate the results by outputting $str.
Call the Trim() method with a single parameter
You can also remove characters other than spaces with Trim() by using parameters. For example, to read data containing strings wrapped in single quotes, remove the quotes by including a single quote as a parameter using the following code.
$str = "' string '"
$str
Write-Host "Length: $($str.Length)"
$str.Trim("'")
Write-Host "Trimmed Length: $($str.Trim("'").Length)"
Here, executing Trim() doesn't remove spaces from the string because Trim() only removes the characters passed in the parameter, which didn't include spaces.

Call the Trim() method with multiple parameters
You can also pass an array of characters to the Trim() method to remove all instances of any of those characters from the start and end of a string. The method keeps removing characters until it encounters a character not included in the parameters.
Continuing with the previous example, the following code removes both single quotes and spaces from the string by including both characters in the parameter.
$str = "' string '"
$str
Write-Host "Length: $($str.Length)"
$str.Trim("' ")
Write-Host "Trimmed Length: $($str.Trim("' ").Length)"
As mentioned above, the Trim() parameters accept characters, but this code passes Trim() a string, as shown in Figure 3.

Here, Trim() converts the string into a character array. In PowerShell, this is simply a matter of typecasting using [char[]]'string'.

To see the parameters for Trim(), you can call the method without any parentheses using the command 'str'.Trim, as shown in Figure 5.

Remove leading and trailing characters with TrimStart() and TrimEnd()
The methods TrimStart() and TrimEnd() are handy for use cases that involve trimming only one end of a string, rather than both.
To see how the two methods work, run the following code.
$str = "'string'"
$str
$str.TrimStart("'")
$str.TrimEnd("'")
As shown in Figure 6, TrimStart() removes the single quote from the beginning, and TrimEnd() removes it from the end.

Practical use cases for the Trim() method
Now that you know how to use the variations of Trim(), take a look at a couple of practical examples.
Writing user creation scripts
A common use case for Trim() involves dealing with input, especially user input. For example, trimming names is useful when writing user creation scripts that accept user input, either directly or from a spreadsheet.
This is especially important if you're passing first and last names to generate usernames. As an example, say HR has sent you a spreadsheet with employees' first and last names, among other data, and your company uses the format firstname.lastname for usernames. Figure 7 shows the imported data that HR provided in the spreadsheet.

In the imported data, the last name has a space at the beginning -- a common problem with copying and pasting. Attempting to create the sAMAccountName or userPrincipalName in Active Directory using the original data will result in the output shown in Figure 8.

Now imagine doing that on a bulk import of a large group of employees from an acquisition, then explaining to executives what went wrong! A simple use of Trim() would have prevented the error.
Working with paths
Another common use for Trim() is when working with paths, especially as parameters. In such cases, using Trim() can eliminate the need to write regular expressions to accept paths with or without a separator at the end.
For example, the following two paths are both valid (assuming the folder in question exists):
- C:\tmp\folder
- C:\tmp\folder\
Likewise, the following web paths are both valid:
- https://domain.com/path/
- https://domain.com/path
But what if you want to join multiple paths? You could join the paths with a slash and hope that a potential double slash doesn't break things, or you could check whether the path ends with a slash and only add one if it doesn't.
Alternatively, you could simply use Trim() to remove any trailing slashes, then add any necessary slash before constructing the new directory path. In the following example, the function takes a base path as a parameter and then adds a subfolder.
Function Do-Things {
param (
[string]$Path
)
New-Item -LiteralPath "$($Path.TrimEnd('/\'))
ew\folder" -ItemType Directory
}
With this code, it doesn't matter whether the $Path parameter has a trailing slash because the function handles both cases. If the original path contains a slash, Trim() removes it; if it doesn't, it won't matter.
If you are working on Windows, multiple directory separators don't invalidate the path, as shown in Figure 9.

However, using multiple separators on a web path is not valid.