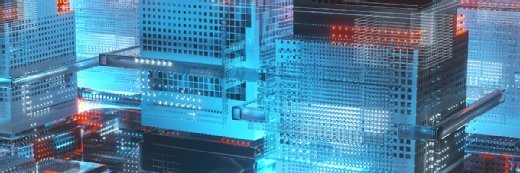
Getty Images/iStockphoto
Manage Kubernetes clusters with PowerShell and kubectl
Learn how to use the Kubernetes tool kubectl in PowerShell, including setting up aliasing and tab-completion, parsing JSON output and searching logs with the Select-String cmdlet.
When it comes to managing a Kubernetes cluster, kubectl is the de facto command-line tool. Whichever platform or shell you use, there's a version of kubectl for you.
The only real difference between using kubectl in PowerShell vs. Bash, for example, is which tools are available to parse the output. Tools such as grep, awk or jq work, but you can also parse JSON output inside PowerShell using built-in cmdlets and a little know-how.
In this walkthrough, learn to set up aliasing and autocompletion in kubectl, parse kubectl's JSON output and use PowerShell's Select-String cmdlet to search log output.
How to set up kubectl in PowerShell
To follow along with this tutorial, first make sure that you've installed kubectl.
Kubectl aliasing in PowerShell
A good initial step when setting up PowerShell to manage Kubernetes on a new device is to alias kubectl commands. Aliasing in PowerShell is the technique of using an alternate name to reference a command.
For example, to avoid typing kubectl over and over, you can alias kubectl to k. Using the New-Alias cmdlet, set the alias name with the -Name parameter and establish the target command's value with the -Value parameter:
New-Alias -Name 'k' -Value 'kubectl'
After setting up this alias, you can type k instead of kubectl, saving six characters each time you make a call to Kubernetes. To use this alias across launch sessions, add it to your PowerShell profile, which maps the alias for you automatically.
Kubectl autocompletion in PowerShell
Next, use the following command to set up autocompletion for kubectl commands in the PowerShell console:
kubectl completion powershell | Out-String | Invoke-Expression
Add autocompletion to your PowerShell profile with the following command:
kubectl completion powershell | Out-File $PROFILE -Append
After completing this step, type k or kubectl and press the Tab key to cycle through the available commands.
Parsing kubectl's JSON output
One of the easiest ways to handle data from kubectl in PowerShell is to set the output type to JSON and then pipe it to ConvertFrom-Json. Kubectl uses the -o parameter to change output type. Pass json to this parameter to get JSON data.
For example, the following command gets all pods and converts them to PowerShell objects:
k get pod -o json | ConvertFrom-Json
Running this command results in the output shown in Figure 1.

To show detailed output about pods, run the following code to examine the items property:
(k get pod -o json | ConvertFrom-Json).items

Setting up JSON output in kubectl enables you to use PowerShell cmdlets such as Where-Object, Select-Object and Format-Table. For example, to find all pods that are less than a day old, use the following command:
(k get pod -o json | ConvertFrom-Json).items | ?{$_.metadata.creationTimestamp -gt (get-date).AddDays(-1)}

Similarly, execute the following command to find all pods associated with a specific volume:
(k get pod -o json | ConvertFrom-Json).items | ?{$_.spec.volumes.name -contains 'certs'}
Using the Select-String cmdlet in PowerShell
With the Select-String cmdlet, you can search text in PowerShell using regular expressions. And fortunately for those who aren't familiar with regular expressions, Select-String can also search for specific strings that are not regular expressions.
As with kubectl, it's helpful to use an alias for Select-String. In this case, PowerShell already offers a built-in alias: sls.
Select-String is particularly useful for searching output from the logs command. For example, run the following code to search for the word "error" in the logs command output, where $pod is the name of one of your pods:
k logs $pod | sls error

The output shows Select-String's highlighting capability. This comes in handy when searching for regular expressions because it highlights exactly what was matched.
For example, the following command highlights all globally unique identifiers (GUIDs) in log output using Select-String and a regular expression to capture GUIDs.
k logs $pod --tail 10 | sls '[a-f0-9]{8}-([a-f0-9]{4}-){3}[a-f0-9]{12}'
There are additional considerations to keep in mind when searching log output for a string that contains special characters, such as a specific URL or portion of a URL.
Slashes and periods are special characters in regular expressions, and you may come across other special characters without recognizing them. To avoid needing to remember which characters in regular expressions are special characters, escape all potential special characters with the PowerShell command [regex]::Escape().
To demonstrate, use the following command to search log output for the string https://localhost/:
k logs $pod | sls ([regex]::Escape('https://localhost/'))
The target string is highlighted in the output, shown in Figure 5.

Kubernetes and PowerShell are each powerful tools in their own right, and using them together lets you work smarter rather than harder. Even with all the tutorials on using Bash commands to improve kubectl use, it's worth exploring the advantages of PowerShell objects and the PowerShell pipeline for kubectl.